Batch / VBS hybrid solution
Tested with Windows XP SP3, Vista SP2 and Windows 7 SP1. It's a specially crafted batch script with an embedded VB Script which does the actual job. This way you get a script which should be compatible with any operating system released after XP SP2. The main credit goes to jeb and dbenham who come up with (and refined) the hybrid technique used here.
REM^ &@echo off>nul
REM^ &if "%~2" == "" exit /b 2
REM^ &pushd "%~2"
REM^ &cscript //e:vbscript //nologo "%~f0" "%~1"
REM^ &popd
REM^ &exit /b
Dim stream, text, lines, fso
Set stream = CreateObject("ADODB.Stream")
stream.Open
stream.Type = 2
stream.Charset = "utf-8"
stream.LoadFromFile Wscript.Arguments(0)
text = stream.ReadText
stream.Close
lines = Split(text, vbCrLf)
Set fso = CreateObject("Scripting.FileSystemObject")
For i = 0 To UBound(lines)
fso.CreateFolder(lines(i))
Next
Instructions
Copy and paste the script above into Notepad or any other plain text editor (e.g. Notepad++).
Save it as CreateFolders.cmd
or whatever name you want. Just make sure to keep the .cmd
extension.
Open a command prompt and navigate to the folder where you saved the file:
cd /d "X:\Folder\containing\CreateFolders.cmd"
Run the batch script by specifying the list file and the destination folder as the first and second parameter, respectively:
CreateFolders.cmd "X:\Some\folder\Export.txt" "X:\Destination\folder"
Screenshots
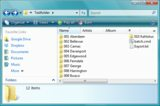
References
PowerShell solution
The following PowerShell script replicates the solution above. It accepts two parameters, the first being the list file (which is assumed to be saved as UTF-8), and the second is the destination folder. Tested with Windows XP SP3, Vista SP2 and Windows 7 SP1.
Note Windows PowerShell 2.0 is bundled with Windows 7, but needs to be manually installed in XP/Vista. As for Windows XP, you need to have .NET Framework 2.0 SP1/SP2 installed, or .NET Framework 3.5 SP1, which include .NET Framework 2.0 SP2. Windows 8 and Windows 8.1 include PowerShell 3.0 and 4.0, respectively.
if ($args.Count -gt 1)
{
$file=$args[0];
$dest=$args[1];
Get-Content $file -Encoding UTF8 | %{ md "$dest\$_" >$null; }
}
Instructions
Copy and paste the script above into Notepad or any other plain text editor (e.g. Notepad++).
Save it as CreateFolders.ps1
(or any other name, as long as you keep the proper extension).
To run the script you can launch PowerShell and then either:
Navigate to the actual path, and then execute it:
cd "C:\Some folder"
& ".\CreateFolders.ps1" "X:\Some\folder\Export.txt" "X:\Destination\folder"
Execute it by specifying the full path directly:
& "C:\Some folder\CreateFolders.ps1" "X:\Some\folder\Export.txt" "X:\Destination\folder"
&
is the call operator.
As an alternative you can start it from a regular command prompt:
powershell -ExecutionPolicy Bypass -NoLogo -NoProfile -File "C:\Some folder\CreateFolders.ps1" "X:\Some\folder\Export.txt" "X:\Destination\folder"
References
Previous (kind of working) solutions
Warning! Do not use them unless you known in advance the targeted system, and the data you're dealing with. If you still want to, make sure they work as expected.
Batch script
The following script will loop through all the lines of the list file and create as many folders, naming them after the actual line content. The script accepts two parameters: the first one is the path where the list is stored, which is hard-coded as Export.txt
(feel free to put a different file name, but avoid spaces); the latter is the destination folder.
@echo off
setlocal
REM make sure there are enough parameters
if "%~2" == "" exit /b 2
REM set the working directory
pushd "%~1"
REM loop through the list and create the folders
for /f "delims=" %%G in (Export.txt) do (md "%~2\%%~G")
REM restore the working directory and exit
popd
endlocal & exit /b
Known limitations
- Either ASCII or ANSI input files only.
Batch script - alternate UTF-8 version
This script is similar to the above one, but in this case the text file is not read directly but parsed through the type
command output. The chcp
is required first in order to change the encoding to UTF-8. In this case the list is not hard-coded but has to be specified as part of the first parameter. The second parameter is the destination folder.
@echo off
setlocal
REM make sure there are enough parameters
if "%~2" == "" exit /b 2
REM set the working directory
pushd "%~2"
REM set the list file
set file="%~1"
REM set the encoding to UTF-8
chcp 65001 >nul
REM loop through the list and create the folders
for /f "delims=" %%G in ('type %file%') do (md "%%~G")
REM restore the working directory and exit
popd
endlocal & exit /b
Known limitations
- On English locales, the
chcp 65001
command will halt the batch script execution, thus skipping any other command.
I realize this is probably way to late for you, but the reason some of the names doesn't work correctly is because of the file's encoding. I downloaded your list of names, opened it in Notepad++ and clicked "Encoding" then "Convert to ANSI" and it worked right away. – Martin – 2016-02-02T18:55:35.953
Do you want the folder name to include the digits too? – and31415 – 2014-02-27T14:13:17.710
That is correct. – Samir – 2014-02-27T14:14:59.077
The list above was just a short example. My actual list of folders to create is 245, from 001 to 245. That's a lot of folders! It doesn't even end there. I will have to create a second, a third and a fourth parent folder and then create subfolders within these, ranging from 001 to 120, 001 to 200, etc. and ad infinitum. So I will re-use these scripts several times. Since I have the names that these folders need to have stored in a text file, this will greatly help me load off some of the manual work I need to do. So I greatly appreciate this guys. Thanks! – Samir – 2014-02-27T20:50:29.957
I've since updated my original answer to provide additional information to chew on. – and31415 – 2014-03-01T15:54:28.777