I would like to export my private key from a Java Keytool keystore, so I can use it with openssl. How can I do that?
-
A nice reference https://8gwifi.org/docs/jks.jsp – anish Oct 06 '18 at 05:32
5 Answers
Use Java keytool to convert from JKS to P12...
Export from keytool
's proprietary format (called "JKS") to standardized format PKCS #12:
keytool -importkeystore \
-srckeystore keystore.jks \
-destkeystore keystore.p12 \
-deststoretype PKCS12 \
-srcalias <jkskeyalias> \
-deststorepass <password> \
-destkeypass <password>
...then use openssl to export from P12 to PEM
Export certificate using openssl
:
openssl pkcs12 -in keystore.p12 -nokeys -out cert.pem
Export unencrypted private key:
openssl pkcs12 -in keystore.p12 -nodes -nocerts -out key.pem
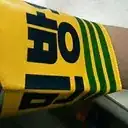
- 103
- 4

- 2,598
- 3
- 17
- 17
-
1
-
8"No DES", i.e. to not encrypt the private key that will be saved to `key.pem`. – Jaime Hablutzel Jun 10 '15 at 17:14
-
4I did as described in this answer, but somehow my exported private key is just an empty file? What gives? – Stijn de Witt Jun 28 '15 at 18:32
-
Just take a look at the output of `openssl pkcs12 -in keystore.p12`, what is present in that output? – Jaime Hablutzel Jun 28 '15 at 23:32
-
`key.pem` starts with `Bag Attributes...`, which my appliances didn't like. I had to add an extra command at the end: `openssl rsa -in -key.pem -out key2.pem`, so that the key would be in the PEM format my appliance required. – Moshe Dec 24 '19 at 01:30
-
use -legacy if you get "digital envelope routines:inner_evp_generic_fetch:unsupported:crypto" – reto Dec 09 '21 at 16:05
Since Java 6, you can import/export private keys into PKCS#12 (.p12
) files using keytool
, with the option -importkeystore
(not available in previous versions).
For example:
keytool -importkeystore -srckeystore existing-store.jks -destkeystore new-store.p12 -deststoretype PKCS12
The PKCS12
keystore type is also supported as a standard keystore type in the default Oracle/Sun security provider.
-
1This feels a bit like the old regex adage, where you now have 2 problems. What do you do with this PCKS12 keystore you have? I'd prefer the below answer as it explains both how to export to PCKS12, and also how to actually get the private key from it. – Scott Sep 18 '17 at 21:19
-
Not entirely made clear elsewhere: convert your java keystore to the newer PKS12 format, instead of the older format. And then you can much more robustly deal with moving private keys around. keytool works fine with keystores in PKS12 format thereafter. Use the -importkeystore invocation given above, and then use the output file everywhere you used it with the original jks file. Or just copy it on top of your old (previously BACKED UP just in case) .jks file. – Robin Davies Mar 19 '20 at 12:30
Try "Keystore Explorer"
I agree with Bruno. Keytool is ultimate tool when dealing with Java keystore, but there is one fancy and quite powerful free tool: Keystore explorer
I use it a lot and never had a need for something else.
-
3Hmm, a Sourceforge site - aren't they known for injecting malware into downloads? Not sure I'd use a tool from Sourceforge on my keys. – gub Jan 09 '16 at 15:37
-
This tool, at least on the Mac, is full of null pointer errors. – Bruno Bronosky Feb 16 '16 at 18:24
-
3KeyStore Explorer has moved to GitHub a while ago. New website is http://keystore-explorer.org – Omikron Feb 24 '16 at 01:32
-
Wish it had a CLI (cmd line interf) thi. The author toyed w/ the idea, bu decided against it. Shame, it does solve many of "keytool"s shortcomings like additionally not supporting access/export of symmetric ("secret") keys. These cannot be converted to PKCS12 (format doesn't support symm keys). There is the JDK "KeyStore" API tho, as @cjbooms pointed out; heck, write ur own "keytool" (that's what they did)... – galaxis Jun 10 '19 at 21:02
If anyone finds themselves here trying to get a private key out of a JCEKS type keystore, I found that the keytool and openssl instructions described in other answers did not work. I had to use the below Java class to get the key out.
import sun.misc.BASE64Encoder;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileWriter;
import java.security.*;
public class ExportPrivateKey
{
private File keystoreFile;
private String keyStoreType;
private char[] keyStorePassword;
private char[] keyPassword;
private String alias;
private File exportedFile;
public void export() throws Exception {
KeyStore keystore = KeyStore.getInstance(keyStoreType);
BASE64Encoder encoder = new BASE64Encoder();
keystore.load(new FileInputStream(keystoreFile), keyStorePassword);
Key key = keystore.getKey(alias, keyPassword);
String encoded = encoder.encode(key.getEncoded());
FileWriter fw = new FileWriter(exportedFile);
fw.write("---BEGIN PRIVATE KEY---\n");
fw.write(encoded);
fw.write("\n");
fw.write("---END PRIVATE KEY---");
fw.close();
}
public static void main(String args[]) throws Exception {
ExportPrivateKey export = new ExportPrivateKey();
export.keystoreFile = new File(args[0]);
export.keyStoreType = args[1];
export.keyStorePassword = args[2].toCharArray();
export.alias = args[3];
export.keyPassword = args[4].toCharArray();
export.exportedFile = new File(args[5]);
export.export();
}
}
Usage:
javac ExportPrivateKey.java
java ExportPrivateKey <path_to_keystore> JCEKS <keystore_password> “<key_alias>” <key_password> <output_file_name>

- 61
- 1
- 1
-
1For any `keytool` command to use a format other than JKS you must specify it; for `-importkeystore` add `-srcstoretype jceks`. – dave_thompson_085 Sep 30 '16 at 07:14
-
1That did exactly what I wanted. Thank you. I created the key: `keytool -v -keystore output.p12 -genseckey -storetype PKCS12 -keyalg AES -alias new_aes_key -keysize 256` then I was able to extract the key: `java ExportPrivateKey output.p12 pkcs12 password new_aes_key password new.pem` – user1683793 May 02 '17 at 23:52
There is a format that allows the moving of private keys is called PKCS#12. This format came later in the evolution of PKI certificates and related keystores as the need evolved. If you consider the chain of trust issues created by accessing and transporting the private key you can see why it was not included in the initial features but rather came after pressure by operational need. This is the core reason for the challenge.
Java keystores were one of the initial users of the PKCS#12 format but as importers not exporters. It appears the security design of Java keystores still does not support exporting private keys as a standard feature. Again, there are good security reasons for this fact. That said, private routines have been written as referenced here: http://www.startux.de/index.php/java/44-dealing-with-java-keystoresyvComment44
If at all possible I would consider creating a new keystore in OpenSSL and new keys rather than trying to pry out the private key from the Java keystore. By opening the Java keystore and extracting the private key one is moving beyond the designed security features. The export PKCS#12 feature has been desired for many years but still is not supported in Java. My thinking is that is for very good cryptologic reasons thus I would be leary of taking that step unless it was absolutely necessary.

- 3,377
- 15
- 22
-
-
1OK I will edit to explain I am concerned with exposing the private key. – zedman9991 May 13 '11 at 20:32
-
3export to PKCS#12 (via the PKCS12 keystore type) has been supported by `keytool` since the release of Java 6. – Bruno May 13 '11 at 21:21
-
4I'm not sure if this concern about exporting the private key (or converting its container) is really justified. It makes perfect sense to re-use the same private key if it matches a certificate that has been signed by a CA, for example (otherwise, the cert would have to be re-issued too), which may happen when changing the implementation of the server (e.g. Java-based server to Apache HTTPD or a reverse proxy). Converting from one format to another doesn't mean you have to be careless. – Bruno May 13 '11 at 21:45
-
This answer is wrong: Java's `keytool` _does_ allow exporting the private key, as the other answers explain. – sleske Feb 09 '16 at 13:19