IBM PC DOS, 8088 assembly, 54 35 bytes
-19 bytes using the difference method
ac2c 41d0 d8d7 7206 51b1 04d2 e859 240f 2c03 02e0 e2ea 3534 4527 4125 1303 1462 4523 13
Unassembled:
; compare dashes and dots in a morse code string
; input:
; I: pointer to input string (default SI)
; IL: length of input string (default CX)
; TBL: pointer to data table (default BX)
; output:
; Sign/OF flags: Dot-heavy: SF == OF (JGE), Dash-heavy: SF != OF (JL)
MORSE_DD MACRO I, IL, TBL
LOCAL LOOP_LETTER, ODD
IFDIFI <I>,<SI> ; skip if S is already SI
MOV SI, I ; load string into SI
ENDIF
IFDIFI <IL>,<CX> ; skip if IL is already CX
MOV CX, IL ; set up loop counter
ENDIF
IFDIFI <TBL>,<BX> ; skip if TBL is already BX
MOV BX, OFFSET TBL ; load letter table into BX
ENDIF
LOOP_LETTER:
LODSB ; load next char from DS:SI into AL, advance SI
;AND AL, 0DFH ; uppercase the input letter (+2 bytes)
SUB AL, 'A' ; convert letter to zero-based index
RCR AL, 1 ; divide index by 2, set CF if odd index
XLAT ; lookup letter in table
JC ODD ; if odd index use low nibble; if even use high nibble
PUSH CX ; save loop counter (since SHR can only take CL on 8088)
MOV CL, 4 ; set up right shift for 4 bits
SHR AL, CL ; shift right
POP CX ; restore loop counter
ODD:
AND AL, 0FH ; mask low nibble
SUB AL, 3 ; unbias dash/dot difference +3 positive
ADD AH, AL ; add letter difference to sum (set result flags)
LOOP LOOP_LETTER
ENDM
TBL DB 035H, 034H, 045H, 027H, 041H, 025H, 013H, 003H, 014H, 062H, 045H, 023H, 013H
Explanation
Implemented in Intel/MASM syntax as a MACRO (basically a function), using only 8088 compatible instructions. Input as uppercase string (or +2 bytes to allow mixed-case), output Truthy/Falsy result is SF == OF
(use JG
or JL
to test).
The letter difference table values are stored as binary nibbles, so only takes 13 bytes in total.
Original (54 bytes):
; compare dashes and dots in a Morse code string
; input:
; I: pointer to input string (default SI)
; IL: length of input string (default CX)
; TBL: pointer to data table
; output:
; Carry Flag: CF=1 (CY) if dot-heavy, CF=0 (NC) if dash-heavy
MORSE_DD MACRO I, IL, TBL
LOCAL LOOP_LETTER
IFDIFI <I>,<SI> ; skip if S is already SI
MOV SI, I ; load string into SI
ENDIF
IFDIFI <IL>,<CX> ; skip if IL is already CX
MOV CX, IL ; set up loop counter
ENDIF
MOV BX, OFFSET TBL ; load score table into BX
XOR DX, DX ; clear DX to hold total score
LOOP_LETTER:
LODSB ; load next char from DS:SI into AL, advance SI
;AND AL, 0DFH ; uppercase the input letter (+2 bytes)
SUB AL, 'A' ; convert letter to zero-based index
XLAT ; lookup letter in table
MOV AH, AL ; examine dot nibble
AND AH, 0FH ; mask off dash nibble
ADD DH, AH ; add letter dot count to total
PUSH CX ; save loop counter (since SHR can only take CL)
MOV CL, 4 ; set up right shift for 4 bits
SHR AL, CL ; shift right
POP CX ; restore loop counter
ADD DL, AL ; add letter dash count to total
LOOP LOOP_LETTER
CMP DL, DH ; if dot-heavy CF=1, if dash-heavy CF=0
ENDM
; data table A-Z: MSN = count of dash, LSN = count of dot
TBL DB 011H, 013H, 022H, 012H, 001H, 013H, 021H, 004H, 002H
DB 031H, 021H, 013H, 020H, 011H, 030H, 022H, 031H, 012H
DB 003H, 010H, 012H, 013H, 021H, 022H, 031H, 022H
Explanation
Implemented in Intel/MASM syntax as a MACRO (basically a function), using only 8088 compatible instructions. Input as string, output Truthy/Falsy result in Carry Flag. Score table contains the number of dashes and dots per letter.
Input is upper case. Add 2 bytes to take lower or mixed case.
Example Test Program (as IBM PC DOS standalone COM executable)
SHR SI, 1 ; point SI to DOS PSP
LODSW ; load arg length into AL, advance SI to 82H
MOV CL, AL ; set up loop counter in CH
DEC CX ; remove leading space from letter count
MORSE_DD SI, CX, TBL ; execute above function, result is in CF
MOV DX, OFFSET F ; default output to "Falsy" string
JA DISP_OUT ; if CF=0, result is falsy, skip to output
MOV DX, OFFSET T ; otherwise CF=1, set output to "Truthy" string
DISP_OUT:
MOV AH, 09H ; DOS API display string function
INT 21H
RET
T DB "Truthy$"
F DB "Falsy$"
Example Output:
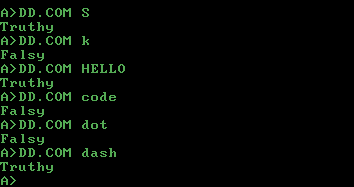
Download test program DD.COM
Or Try it Online!
I'm not aware of an online TIO to direct link to a DOS executable, however you can use this with just a few steps:
- Download DD.COM as a ZIP file
- Go to https://virtualconsoles.com/online-emulators/DOS/
- Upload the ZIP file you just downloaded, click Start
- Type
DD Hello
or DD code
to your heart's content
Related – Bassdrop Cumberwubwubwub – 2019-03-11T16:35:33.823
4Can we return a value above 0 for dotheavy and a negative value for dash-heavy? – Embodiment of Ignorance – 2019-03-11T20:20:18.977
@EmbodimentofIgnorance That works for me, as long as you specify it in your post. I don't think it usually passes the truthy falsy test but it feels like a good solution in this case so I will allow it – Bassdrop Cumberwubwubwub – 2019-03-12T08:38:06.700