I just had the need for the same thing OP is asking and after searching on Google and reading the answers, none of them provided what I think the OP and I are looking for.
The problem here is that one may map a network share to Drive Y
whereas someone else in the organization may have the same network share mapped as Drive X
; therefore, sending a link such as Y:\mydirectory
may not work for anyone else except me.
As the OP explains, Explorer does show the actual path in the Explorer bar, but you cannot copy it (typing is tedious and prone to errors, so this is not an option) even if you choose copy as path
from the context menu:
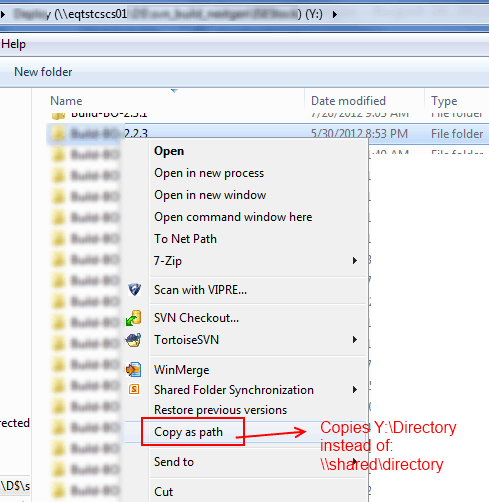
So the solution I came up with (by copying someone else's code) was a little C# program that you can call from a context menu in Explorer and will allow you to translate the Mapped drive letter to the actual UNC path
.
Here's the code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace Utils
{
//This is the only piece of code I wrote
class Program
{
[STAThread]
static void Main(string[] args)
{
//Takes the parameter from the command line. Since this will
//be called from the context menu, the context menu will pass it
//via %1 (see registry instructions below)
if (args.Length == 1)
{
Clipboard.SetText(Pathing.GetUNCPath(args[0]));
}
else
{
//This is so you can assign a shortcut to the program and be able to
//Call it pressing the shortcut you assign. The program will take
//whatever string is in the Clipboard and convert it to the UNC path
//For example, you can do "Copy as Path" and then press the shortcut you
//assigned to this program. You can then press ctrl-v and it will
//contain the UNC path
Clipboard.SetText(Pathing.GetUNCPath(Clipboard.GetText()));
}
}
}
}
And here's the Pathing
class definition (I'll try to find the actual source as I can't remember where I found it):
public static class Pathing
{
[DllImport("mpr.dll", CharSet = CharSet.Unicode, SetLastError = true)]
public static extern int WNetGetConnection(
[MarshalAs(UnmanagedType.LPTStr)] string localName,
[MarshalAs(UnmanagedType.LPTStr)] StringBuilder remoteName,
ref int length);
/// <summary>
/// Given a path, returns the UNC path or the original. (No exceptions
/// are raised by this function directly). For example, "P:\2008-02-29"
/// might return: "\\networkserver\Shares\Photos\2008-02-09"
/// </summary>
/// <param name="originalPath">The path to convert to a UNC Path</param>
/// <returns>A UNC path. If a network drive letter is specified, the
/// drive letter is converted to a UNC or network path. If the
/// originalPath cannot be converted, it is returned unchanged.</returns>
public static string GetUNCPath(string originalPath)
{
StringBuilder sb = new StringBuilder(512);
int size = sb.Capacity;
// look for the {LETTER}: combination ...
if (originalPath.Length > 2 && originalPath[1] == ':')
{
// don't use char.IsLetter here - as that can be misleading
// the only valid drive letters are a-z && A-Z.
char c = originalPath[0];
if ((c >= 'a' && c <= 'z') || (c >= 'A' && c <= 'Z'))
{
int error = WNetGetConnection(originalPath.Substring(0, 2),
sb, ref size);
if (error == 0)
{
DirectoryInfo dir = new DirectoryInfo(originalPath);
string path = Path.GetFullPath(originalPath)
.Substring(Path.GetPathRoot(originalPath).Length);
return Path.Combine(sb.ToString().TrimEnd(), path);
}
}
}
return originalPath;
}
}
You build the program and put the executable somewhere in your PC, say for example, in c:\Utils
Now you add a context menu option in Explorer as so:
Regedit and then:
HKEY_CLASSES_ROOT\*\Directory\Shell
Right-click Shell --> New Key --> Name: "To UNC Path"
Right-click To UNC Path --> New Key --> Name: command
Right-click Default entry and select `Modify`
Value Data: c:\Utils\Utils.exe "%1"
You are done. Now you'll see this option when you right-click a directory from a mapped drive:
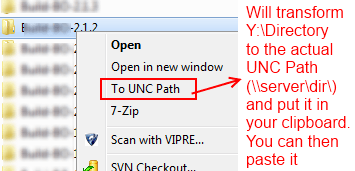
Note
I can provide the executable so you don't have to do the compilation yourself. Simply drop me a note here.
1
If you're running in a locked down corporate environment where you cannot install any third party applications and/or access the registry, then this solution will work ... http://superuser.com/a/704374/46099
– Richard – 2014-02-11T11:52:07.257