I'm trying to write a backup script that can be deployed on many different linux servers, some of which mount network drives as described in /etc/fstab. I want my script to be able to see if any network drives have come unmounted before a backup to ensure that they don't go unsaved. Is there any way to reliably check for this in a bash script?
-
@Iain et al: Just as a point of information, the duplicate answer is OS-specific. This one is not. – Jim L. May 31 '19 at 17:10
2 Answers
Combining the test
command with the -d
flag and checking to see if the mount exists per this question:
#!/bin/bash
mount="/fileserver"
if mountpoint -q "$mount" && test -d /path/to/share; then
cp -ru /path/to/files /. -t /path/to/share
fi
Edited per Michael Hampton's comment.
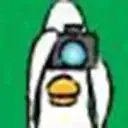
- 1,210
- 3
- 14
- 24
-
1The mountpoint may always exist even if the filesystem is not mounted on it. This doesn't make a good test by itself. – Michael Hampton May 23 '19 at 00:08
-
-
One method that comes to mind is to iterate over the list of mountpoints and see how many files are present under each one. A value of 1 probably means the filesystem isn't mounted (and only the directory itself is present). This strategy won't work if the mountpoints are nested, however. By "nested" I mean mountpoints like:
/mnt/server1/share1
/mnt/server1/share1/share2
/mnt/server1/share1/share2/share3
I have also seen this fail when someone/some process didn't know that a mount wasn't there, and copied files to it anyway, and the files got written to the underlying filesystem, instead of to the filesystem that should have been mounted there.
But if your structure is more "flat" (or if it can be made flat for backup purposes), like:
/mnt/server1/share1
/mnt/server1/share2
/mnt/server2/share3
then:
MNTS="/mnt/server1/share1 /mnt/server1/share2 /mnt/server2/share3"
for DIR in $MNTS; do
N=$(find $DIR | head -10 | wc -l)
if [ $N -eq 1 ]; then
print "%s appears to not be mounted\n" "$DIR"
else
# back it up
fi
done
Another method that might be slightly OS-specific, or at least subject to change if mount
's output format changes, is to do a brute-force check of mount
's output. Assuming that your mount
always uses spaces and never uses tabs, then:
MNTS="/mnt/server1/share1 /mnt/server1/share2 /mnt/server2/share3"
for DIR in $MNTS; do
if ! mount | grep -F " $DIR "; then
print "%s appears to not be mounted\n" "$DIR"
else
# back it up
fi
done

- 645
- 4
- 10
-
I wound up using a solution like this, with the MNTS variable being an environment variable set on each server during customization. – MindlessMutagen May 30 '19 at 15:41