I'm looking for a single inline command to execute /bin/first
and then when it completes execute the following /bin/p1
, /bin/p2
, /bin/p3
in parallel after.
Asked
Active
Viewed 167 times
1

Justin
- 5,008
- 19
- 58
- 82
2 Answers
3
When you start and run commands in the background from a shell script by appending a & to the command, they will run almost completely in parallel because the script won't need to wait until the command completes before executing the next line in the script.
#!/bin/bash
/bin/first
/bin/p1 &
/bin/p2 &
/bin/p3
Note: When the script completes p1 and p2 may still be running in the background!
To alleviate that your script becomes slightly more complex as you'll need to capture the PID's and wait
for them:
#!/bin/bash
/bin/first
/bin/p1 &
PID1=$!
/bin/p2 &
PID2=$!
/bin/p3 &
PID3=$!
for PIDS in $PID1 $PID2 $PID3 ;
do
wait $PIDS
done
That for loop is only needed if you want to do some error handling and check the exit code of the programs you executed in parallel. Otherwise a simple wait
without any arguments will also wait for all child processes to terminate.

HBruijn
- 72,524
- 21
- 127
- 192
-
there a way to have the script only complete once all `p1`, `p2`, `p3` are complete? – Justin Sep 17 '17 at 07:56
-
capture the PID's and use the bash built-in function `wait` function. – HBruijn Sep 17 '17 at 08:22
1
If you need to make sure the output does not mix:
/bin/first
parallel ::: /bin/p1 /bin/p2 /bin/p3
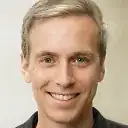
Ole Tange
- 2,836
- 5
- 29
- 45