Are there any off-the-shelf tools that will expose the basic health of a Windows machine (network accessibility, system load, etc.) over HTTP in a machine-readable form that I can access from another host?
2 Answers
If by "off the shelf" you mean "native to the OS", then no*.
The common native methods of gathering Windows performance statistics are Perfmon (TCP 445), WMI (TCP 445) , or SNMP (UDP 161/162).
Are you interested in just using HTTP for the data connection, or actually desiring to run a human-readable webserver on each target Windows machine showing a health dashboard? If you're running HP or Dell servers, that's actually already included in the vendor-provided systems agents, although it's mostly focused on hardware stats, so not really appropriate for VM guests.
- I suppose you could count using WinRM over HTTP(S) and gathering perfmon stats directly...
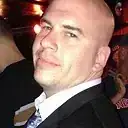
- 35,711
- 3
- 50
- 86
-
I'm open to 3rd-party tools. I don't need the machines to serve the data in a human-readable form, just expose JSON or something over HTTP so other HTTP-only services in my infrastructure can aggregate it. – spiffytech May 19 '17 at 14:06
-
OK, understood. Product recommendations are off-topic per the FAQ, and I don't know of any for this purpose anyway. I think you're on your own, it sounds like you need something fairly specific. Perhaps you can write an agent that could be installed on a local system, perfmon in what you care about, and present that via HTTPS for consumption by you monitoring service. What is your current monitoring service? – mfinni May 19 '17 at 20:45
-
Would be trivial to write a little flask + psutil app. Probably wouldn't be more than 50 lines of code. – Brennen Smith May 20 '17 at 04:38
Alright- not exactly what you were looking for, but I was bored and this is a trivial issue to solve. Here is a little flask application:
#!/usr/bin/env python
import psutil
import flask
import json
from flask import Flask
app = Flask(__name__)
def getStats():
data = {}
data['cpu_percent'] = psutil.cpu_percent(interval=1, percpu=True)
data['memory_virt'] = psutil.virtual_memory()
data['disk_io'] = json.dumps(psutil.disk_io_counters())
data['disk_usage'] = psutil.disk_usage("C:") # double check this, I'm on osx
return json.dumps(data)
@app.route('/')
def main():
return getStats()
if __name__ == "__main__":
app.run(host='0.0.0.0')
Which returns a JSON representation of your CPU, memory, disk IO, and disk usage:
{
"disk_usage":[
371011354624,
273490915328,
97258295296,
73.8
],
"memory_virt":[
17179869184,
5456252928,
68.2,
14814887936,
102600704,
5523148800,
5353652224,
3938086912
],
"disk_io":"[18150220, 10587005, 1112392108544, 834027424256, 9743448, 3448989]",
"cpu_percent":[
20.0,
1.0,
16.0,
0.0,
12.0,
0.0,
16.7,
1.0
]
}
All you need to run this program is
(save the code above to remote-mon.py and open port 5000 in Windows Firewall)
pip install flask psutil
python remote-mon.py
Then you can call against http://{{machine'sIP}}:5000/ for the json object.
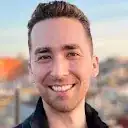
- 1,638
- 7
- 11