If you don't want packet sniffing, I would recommend a powershell script in all the computers testing a secure ldap connection and logging who fails. You could connect remotely to the clients from the Domain Controller or you could make a client side script who logs failures on a fileserver.
The ideia of the script is to simulated a secure ldap connection. It uses .net framework that comes natively on windows 7 sp1 or higher.
In case you want to run remotely from the DC, the script would look like this (requires permission for remote powershell which can be achieved following this article https://www.briantist.com/how-to/powershell-remoting-group-policy/):
Import-Module ActiveDirectory
$domain = "contoso.com"
$user = "Administrator"
$password = "P@ssw0rd"
$IPFilter = "192.168.1.*"
$scriptblock = {
write-host "$(hostname) - " -NoNewLine
try {
$LDAPS = New-Object adsi ("LDAP://$($args[0]):636",$args[1],$args[2],'SecureSocketsLayer')
Write-Host "Secure LDAP Connection succeeded."
} Catch {
Write-Host "Secure LDAP Connection failed." -foregroundcolor red
}
}
$Computers = Get-ADComputer -filter * -Properties IPv4Address | Where{ $_.IPv4Address -like $IPFilter}
foreach($Computer in $Computers)
{
try {
$session = New-PSSession $Computer.Name -ErrorAction Stop
Invoke-Command -Session $session -ScriptBlock $scriptblock -ArgumentList $domain,$user,$password
}catch{
Write-Host "Connection to $($Computer.Name) failed." -foregroundcolor red
}
}
Or if you want a local script which logs into a remote server:
$domain = "contoso.com"
$user = "Administrator"
$password = "P@ssw0rd"
$LogFile = "\\fileserver\logs\ldapconnection.log"
try {
$LDAPS = New-Object adsi ("LDAP://$domain:636",$user,$password,'SecureSocketsLayer')
"$(hostname) - Secure LDAP Connection succeeded." | Out-File $LogFile -Append
} Catch {
"$(hostname) - Secure LDAP Connection failed." | Out-File $LogFile -Append
}
Output of a remote version execution (red ones are offline clients):
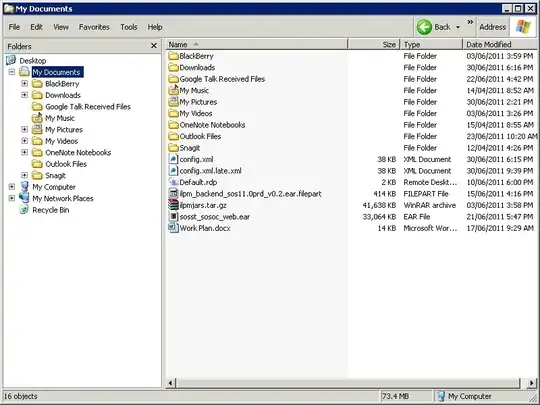