What's the postfix equivalent to sendmail -bp
?
8 Answers
qshape active
will show you the number of emails being sent to each domain and how long they have been in the active queue
qshape deferred
will show you the same but for the deferred queue

- 806
- 1
- 7
- 19
Here's what I use, culled from the postfix mailing list. I removed the author's name, in case he doesn't want it here (you can see it at the source). It only displays totals.
#!/usr/bin/env perl
# postfix queue/s size
# author:
# source: http://tech.groups.yahoo.com/group/postfix-users/message/255133
use strict;
use warnings;
use Symbol;
sub count {
my ($dir) = @_;
my $dh = gensym();
my $c = 0;
opendir($dh, $dir) or die "$0: opendir: $dir: $!\n";
while (my $f = readdir($dh)) {
if ($f =~ m{^[A-F0-9]{5,}$}) {
++$c;
} elsif ($f =~ m{^[A-F0-9]$}) {
$c += count("$dir/$f");
}
}
closedir($dh) or die "closedir: $dir: $!\n";
return $c;
}
my $qdir = `postconf -h queue_directory`;
chomp($qdir);
chdir($qdir) or die "$0: chdir: $qdir: $!\n";
printf "Incoming: %d\n", count("incoming");
printf "Active: %d\n", count("active");
printf "Deferred: %d\n", count("deferred");
printf "Bounced: %d\n", count("bounce");
printf "Hold: %d\n", count("hold");
printf "Corrupt: %d\n", count("corrupt");
EDIT: Fixed a typo on line 26.

- 1,135
- 1
- 14
- 26
-
Great script, and IMHO should be a part of the standard Postfix distribution. Unlike mailq/postqueue returns immediate answer for a queue under duress – Alexander Pogrebnyak Aug 14 '12 at 16:27
-
Just a word of caution on the particular implementation of `count` function. It will fail in Postfix 2.9+ when enable_long_queue_ids=yes'. I think it should not be too hard to fix it for long queue ids. – Alexander Pogrebnyak Aug 15 '12 at 15:58
postqueue -p | tail -n 1
Last line in the postqueue -p
shows how many requests and size:
-- 317788 Kbytes in 11860 Requests.
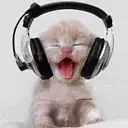
- 231
- 2
- 4
-
1This command executes quickly since it doesn't waste cycles displaying the individual emails in the queue. If just want the total, run this. – Paul Calabro Nov 16 '17 at 17:35
[root@server ~]# time mailq | grep -c '^[0-9A-Z]'
10
real 0m1.333s
user 0m0.003s
sys 0m0.003s
(above result indicating that there are 10 email is queue)

- 61
- 1
- 1
If you don't have qshape
you can install it via the following yum commands:
yum groupinstall perl development
yum install postfix-perl-scripts
qshape prints Postfix queue domain and age distribution information. You can read more about it here:
http://www.postfix.org/QSHAPE_README.html
Example output
% qshape -s hold | head
T 5 10 20 40 80 160 320 640 1280 1280+
TOTAL 486 0 0 1 0 0 2 4 20 40 419
yahoo.com 14 0 0 1 0 0 0 0 1 0 12
extremepricecuts.net 13 0 0 0 0 0 0 0 2 0 11
ms35.hinet.net 12 0 0 0 0 0 0 0 0 1 11
winnersdaily.net 12 0 0 0 0 0 0 0 2 0 10
hotmail.com 11 0 0 0 0 0 0 0 0 1 10
worldnet.fr 6 0 0 0 0 0 0 0 0 0 6
ms41.hinet.net 6 0 0 0 0 0 0 0 0 0 6
osn.de 5 0 0 0 0 0 1 0 0 0 4
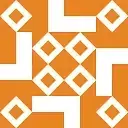
- 7,355
- 16
- 54
- 72
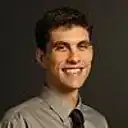
- 589
- 6
- 12
Here is an example.
#!/bin/bash
for q in active bounce corrupt defer deferred flush hold incoming maildrop pid private public saved trace
do
count=$(find /var/spool/postfix/$q ! -type d -print | wc -l)
echo $q $count
done
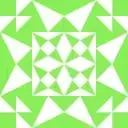
- 105
- 5

- 21
- 1