I am testing nginx and want to output variables to the log files. How can I do that and which log file will it go (access or error).
5 Answers
You can send nginx variable values via headers. Handy for development.
add_header X-uri "$uri";
and you'll see in your browser's response headers:
X-uri:/index.php
I sometimes do this during local development.
It's also handy for telling you if a subsection is getting executed or not. Just sprinkle it inside your clauses to see if they're getting used.
location ~* ^.+.(jpg|jpeg|gif|css|png|js|ico|html|xml|txt)$ {
add_header X-debug-message "A static file was served" always;
...
}
location ~ \.php$ {
add_header X-debug-message "A php file was used" always;
...
}
So visiting a url like http://www.example.com/index.php will trigger the latter header while visiting http://www.example.com/img/my-ducky.png will trigger the former header.

- 2,312
- 2
- 13
- 5
-
36Note that `add_header` will work [on successful requests only](http://wiki.nginx.org/NginxHttpHeadersModule#add_header). Documentation states that it can only be applied to responses with codes 200, 204, 301, 302 or 304. Therefore, it can't be used to debug HTTP errors. – John WH Smith Aug 25 '14 at 14:43
-
42@JohnWHSmith : As marat noted in [this answer](http://serverfault.com/questions/418709/nginx-add-header-for-a-50-page/647552#647552). As of [version 1.7.5](http://nginx.org/en/CHANGES), nginx added an ["always" parameter](http://nginx.org/en/docs/http/ngx_http_headers_module.html) to ```add_header``` which will return the header, no matter what the response code. So for example, ```add_header X-debug-message "A php file was used" always;```, should work even for 500 error code. – yuvilio Sep 22 '15 at 20:24
-
16This doesn't answer the question at all. The guy wants to log to **log file** not to the client. – Avamander Apr 17 '18 at 11:31
-
omg.. so handy. thanks – Shaun Apr 08 '20 at 03:23
-
Doesn't work. I am doing proxy_pass. The header is NOT present in the response. – Arrow_Raider Mar 29 '21 at 16:07
You can return a simple string as HTTP response:
location / {
add_header Content-Type text/plain;
return 200 $document_root;
}
Or use interpolation for watching multiple variables at the same time:
location / {
add_header Content-Type text/plain;
return 200 "document_root: $document_root, request_uri: $request_uri";
}

- 103
- 4
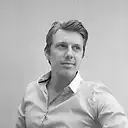
- 1,239
- 11
- 13
-
2
-
Going to the location immediately opens the Save As dialog of my browser (tested on Opera, Chromium). No response whatsoever. – BringBackCommodore64 Mar 16 '18 at 17:27
-
@BringBackCommodore64 Adding a text/html Content-Type header may help. – CuriousGeorge May 09 '19 at 20:26
-
Using [Postman](https://getpostman.com), this works without any additional headers. Thanks! – mehov Oct 07 '19 at 08:10
-
2I want to return multiple variables and solved it like this; `return 200 "xforwardedfor:$proxy_add_x_forwarded_for--remote_addr:$remote_addr--scheme:$scheme--host:$host"; ` – kursat sonmez Nov 27 '20 at 13:15
You can set a custom access log format using the log_format
directive which logs the variables you're interested in.

- 30,036
- 7
- 76
- 121
-
2thanks, and I guess there is no easier way to output one variable by itself? – lulalala Jul 04 '12 at 05:39
-
-
It is possible set the log level in the directive `error_log` to `debug` so you can see the value of the variables and that block that are execute. Example `error_log file.log debug` – Victor Aguilar Jan 10 '17 at 00:55
-
2notice thar empty variables are shown as `-` in the log, but are really empty in the nginx code, you should not check for `-` at any time. This sometimes confuse users. – higuita May 05 '17 at 12:56
Another option is to include the echo module when you build nginx, or install OpenResty which is nginx bundled with a bunch of extensions (like echo.)
Then you can simply sprinkle your configuration with statements like:
echo "args: $args"

- 189
- 1
- 2
-
2When I try this it echos out to a plain text file on the server interrupting the output of the actual page. – JaredMcAteer May 07 '15 at 17:36
-
1
-
1@Gajus Three years later now, and I googled for this, but can't find anything about `echo_log`. Do you know if it ever got out of development? – Randall Oct 30 '20 at 18:14
none of these answer the question as asked (log)
However, @Victor Aguilar - has a comment on this answer, that should be an answer! It says how to log variables, and it works. Thanks!
https://serverfault.com/a/404628/400075
i.e. in /etc/nginx/nginx.conf
error_log /var/log/nginx/error.log debug;
results in the following type of logging:
2021/03/06 15:14:48 [debug] 2550#2550: *1 fastcgi param: "SCRIPT_FILENAME: /usr/lib/cgit/cgit.cgi/something.git/cgit.cgi"
2021/03/06 15:14:48 [debug] 2550#2550: *1 http script copy: "QUERY_STRING"
2021/03/06 15:14:48 [debug] 2550#2550: *1 fastcgi param: "QUERY_STRING: "
2021/03/06 15:14:48 [debug] 2550#2550: *1 http script copy: "REQUEST_METHOD"
2021/03/06 15:14:48 [debug] 2550#2550: *1 http script var: "GET"
2021/03/06 15:14:48 [debug] 2550#2550: *1 fastcgi param: "REQUEST_METHOD: GET"
2021/03/06 15:14:48 [debug] 2550#2550: *1 http script copy: "CONTENT_TYPE"
2021/03/06 15:14:48 [debug] 2550#2550: *1 fastcgi param: "CONTENT_TYPE: "
2021/03/06 15:14:48 [debug] 2550#2550: *1 http script copy: "CONTENT_LENGTH"
2021/03/06 15:14:48 [debug] 2550#2550: *1 fastcgi param: "CONTENT_LENGTH: "
2021/03/06 15:14:48 [debug] 2550#2550: *1 http script copy: "SCRIPT_NAME"
2021/03/06 15:14:48 [debug] 2550#2550: *1 http script var: "/cgit/cgit.cgi/something.git/cgit.cgi"
2021/03/06 15:14:48 [debug] 2550#2550: *1 fastcgi param: "SCRIPT_NAME: /cgit/cgit.cgi/something.git/cgit.
cgi"
2021/03/06 15:14:48 [debug] 2550#2550: *1 http script copy: "REQUEST_URI"
2021/03/06 15:14:48 [debug] 2550#2550: *1 http script var: "/cgit/cgit.cgi/something.git/"
2021/03/06 15:14:48 [debug] 2550#2550: *1 fastcgi param: "REQUEST_URI: /cgit/cgit.cgi/something.git/"
2021/03/06 15:14:48 [debug] 2550#2550: *1 http script copy: "DOCUMENT_URI"
2021/03/06 15:14:48 [debug] 2550#2550: *1 http script var: "/cgit/cgit.cgi/something.git/"
2021/03/06 15:14:48 [debug] 2550#2550: *1 fastcgi param: "DOCUMENT_URI: /cgit/cgit.cgi/something.git/"
2021/03/06 15:14:48 [debug] 2550#2550: *1 http script copy: "DOCUMENT_ROOT"
2021/03/06 15:14:48 [debug] 2550#2550: *1 http script var: "/usr/lib"
2021/03/06 15:14:48 [debug] 2550#2550: *1 fastcgi param: "DOCUMENT_ROOT: /usr/lib"
2021/03/06 15:14:48 [debug] 2550#2550: *1 http script copy: "SERVER_PROTOCOL"
2021/03/06 15:14:48 [debug] 2550#2550: *....

- 41
- 2
-
3not all nginx packages ar buit with --with-debug flag so debug directive is useless in error_log if nginx built with --with-debug (as I see most of nginxes are built without) – Ivan Borshchov May 27 '21 at 11:02