Is there a one-liner that will zip/unzip files (*.zip) in PowerShell?
-
related: [Unzip with PS in Server Core](http://serverfault.com/questions/446884/unzip-file-with-powershell-in-server-2012-core) – Ruben Bartelink Aug 28 '13 at 16:20
11 Answers
This is how you can do it purely from Powershell without any external tools. This unzips a file called test.zip onto the current working directory:
$shell_app=new-object -com shell.application
$filename = "test.zip"
$zip_file = $shell_app.namespace((Get-Location).Path + "\$filename")
$destination = $shell_app.namespace((Get-Location).Path)
$destination.Copyhere($zip_file.items())

- 395
- 3
- 12

- 3,598
- 4
- 25
- 27
-
26use $destination.Copyhere($zip_file.items(), 0x10) for overwriting existing files. 0x4 hides the dialog box, and 0x14 combines these and overwrites and hides the dialog. – Peter Apr 19 '11 at 04:06
-
-
3The line `$destination.Copyhere($zip_file.items())` does the actual unziping. – Jonathan Allen Oct 28 '11 at 22:02
-
2You can parcel the above into a function, if you wanted: `function unzip($filename) { if (!(test-path $filename)) { throw "$filename does not exist" } $shell = new-object -com shell.application $shell.namespace($pwd.path).copyhere($shell.namespace((join-path $pwd $filename)).items()) }` – James Holwell Jan 29 '13 at 11:19
-
6
-
1Agree about accepted. Is not a monstruous code like other PowerShell i see. 5 Lines is just great and is fully related to Powershell not to 3rd party software. – m3nda Dec 10 '13 at 07:04
-
2This fails for me when the zip file contains just a folder (items is empty) – James Woolfenden Aug 19 '14 at 09:16
-
1
-
-
-
1
-
-
how to zip with this method (> is command-prompt, src is a folder): `>$shellapp=new-object -com shell.application >$zippath="test.zip" >$zipobj=$shellapp.namespace((Get-Location).Path + "\$zippath") >$srcpath="src" >$srcobj=$shellapp.namespace((Get-Location).Path + "\$srcpath") >$zipobj.Copyhere($srcobj.items())` – johny why Sep 30 '16 at 18:43
Now in .NET Framework 4.5, there is a ZipFile class that you can use like this:
[System.Reflection.Assembly]::LoadWithPartialName('System.IO.Compression.FileSystem')
[System.IO.Compression.ZipFile]::ExtractToDirectory($sourceFile, $targetFolder)

- 736
- 5
- 9
-
It *definitely* worked - I used it to extract over 400 zip files. I'll check to make sure I don't have 4.5 on here, but it doesn't show up under Microsoft.NET. – GalacticCowboy Feb 13 '13 at 01:13
-
1This *would* be great if there were a simple method to ExtractToDirectory and an option to overwrite all existing files. – James Dunne Apr 02 '13 at 00:43
-
1
-
[Article with example](http://vcsjones.com/2012/11/11/unzipping-files-with-powershell-in-server-core-the-new-way/) based on [related question](http://serverfault.com/questions/446884/unzip-file-with-powershell-in-server-2012-core) – Ruben Bartelink Aug 28 '13 at 16:17
-
3Load the assembly with Add-Type, for example: Add-Type -AssemblyName System.IO.Compression.FileSystem – Mike Sep 19 '13 at 02:39
-
2@JamesDunne - If you don't have other files you need to preserve, could use 'Remove-Item -Recurse $TargetFolder'. Otherwise, what you want can be done, but it would be non-trivial. You would need to open the zip for read, and then walk the zip, deleting any previous target object and unpacking the new one. Lucky for me, the easy solution works. ;) – Mike Sep 19 '13 at 02:44
-
3Be warned that relative file locations will use the *.NET* current directory, *not* the PowerShell one. See [here](http://stackoverflow.com/q/11246068/1394393). It's probably better just to `(Resolve-Path $someDir).Path` the arguments. – jpmc26 Aug 13 '16 at 03:45
-
Oh, I know this is 6 years old, but after trying to find a low-cost commercial (or open source) solution to extract files from a PKZIP SFX, and failing miserably, this solution right here made my day! – Shoeless Aug 30 '18 at 17:28
DotNetZip will allow you to do this from PowerShell. It is not a one-liner, but the library will allow you to write the PowerShell script you need.
You can also use the COM interface, see Compress Files with Windows PowerShell then package a Windows Vista Sidebar Gadget.
Googling "zip powershell" or "unzip powershell" might also turn up useful results.

- 2,319
- 5
- 23
- 24

- 9,064
- 1
- 34
- 41
-
+1 Linked article has useful Tasks unlike the most upvoted answer – Ruben Bartelink Apr 10 '12 at 10:09
-
7-1 for suggesting a Google search. This is the top StackExchange result in a Google search for "unzip powershell" – machine yearning Aug 20 '15 at 09:59
I know this is a very old question, but I just saw it linked on Twitter on figured I'd post a current answer.
PowerShell 5, currently available on Windows 10 or via the Windows Management Framework 5 Production Preview, comes with two built-in cmdlets for 'zipping' and 'unzipping':

- 361
- 3
- 7
-
4It is a *good* thing to provide new information to old questions. ;) +1 – jpmc26 Aug 13 '16 at 03:16
You may wish to check out The PowerShell Community Extensions (PSCX) which has cmdlets specifically for this.

- 5,309
- 1
- 22
- 20
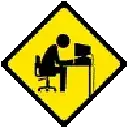
- 1,760
- 10
- 14
-
1+1 PSCX is a great set of add-on cmdlets - I just wish I could pick and choose more which ones I want and which I don't. It seems to be changing a whole lot in my Powershell instance.... – marc_s Jun 12 '09 at 07:48
-
I've come across this because I actually want to automate the PSCX installation if I can for some coworkers. Trying it now to see what sort of issues I run into – jcolebrand Apr 27 '11 at 15:02
I also like Info-ZIP (the Zip engine found in most other Zip utilities) and 7-Zip, another favorite which has both a GUI and command line Zip utility. The point being, there are some good command-line utilities that will work for most PowerShell tasks.
There are some tricks to running command line utilities that were not built with PowerShell in mind:
Running an executable that starts with a number in the name, preface it with an Ampersand (&).
&7zip.exe
Wrap each token, the utility is expecting to see from the command line, in quotes.
&"c:\path with space\SomeCommand.exe" "/parameter2" "/parameter2" "parameter2's Value" "Value2 `" with a quote"
Try this:
zip filename.zip (Get-ChildItem somepath\*)
Or even:
zip filename.zip (Get-Content ListOfFiles.txt)

- 1,620
- 5
- 26
- 38
I find the simplest solution to just use infozip binaries which I have used for years and use in a UNIX environment.
PS> zip -9r ../test.zip *
PS> cd ..
PS> unzip -t test.zip Archive: test.zip
testing: LinqRepository/ OK
testing: LinqRepository/ApplicationService.cs OK
testing: LinqRepository/bin/ OK
...
No errors detected in compressed data of test.zip.
It would be straighforward to put a powershell wrapper around the text output but in practice I never need that so I haven't bothered.

- 860
- 5
- 8
James Holwell I like your answer but I expanded it a little bit
# Example
#unzip "myZip.zip" "C:\Users\me\Desktop" "c:\mylocation"
function unzip($fileName, $sourcePath, $destinationPath)
{
$shell = new-object -com shell.application
if (!(Test-Path "$sourcePath\$fileName"))
{
throw "$sourcePath\$fileName does not exist"
}
New-Item -ItemType Directory -Force -Path $destinationPath -WarningAction SilentlyContinue
$shell.namespace($destinationPath).copyhere($shell.namespace("$sourcePath\$fileName").items())
}

- 121
- 3
The ionic approach rocks:
https://dotnetzip.codeplex.com/wikipage?title=PS-Examples
supports passwords, other crypto methods, etc.
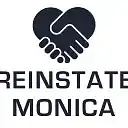
- 5,347
- 9
- 54
- 80
I've created a PowerShell 2.0 compatible module that uses the native Windows OS commands to zip and unzip files in a synchronous manner. This works on older OSs, like Windows XP, and does not require .Net 4.5 or any other external tools. The functions will also block script execution until the files have all been zipped/unzipped. You can find more information and the module on my blog here.

- 303
- 1
- 5
- 9