In windows batch scripts, how can I iterate over all drive letters which correspond to physical volumes or mapped shares?
7 Answers
Also going to suggest a language switch, but to Powershell instead of VBS.
Get-PSDrive -PSProvider FileSystem
It's the wave of the future...

- 11,124
- 1
- 29
- 36
-
This also yields other drives that don't have anything to do with non-PowerShell things. On my system, `Home:` is a PSDrive to my home directory, for example. You're getting more back than needed here. – Joey Apr 18 '10 at 13:04
-
1`gwmi win32_logicaldisk | where {$_.DriveType -eq 3}` for physical drives, if you want only those with drive letters (possible to not have any mount point, or could be mounted to a folder) add `| where {$_.DeviceID -like "*:"}`. for network drives just change the original where statement to `where {$_DriveType -eq 4}` (3 = Local disk, 4 = Network disk). PowerShell is absolutely awesome once you get the hang of these crazy commands. If you list exactly what you're trying to do, I can elaborate more. – Chris S Apr 18 '10 at 13:24
-
Another powershell option that doesn't pull back powershell-specific drives: `[System.IO.DriveInfo]::GetDrives()` – MattB Apr 19 '10 at 13:05
Maybe there are better tools now, but there used to be the fsutil
command (WinXP).
fsutil fsinfo drives
this returns all the drives in the system.

- 5,384
- 2
- 23
- 14
-
2Vista: "The FSUTIL utility requires that you have administrative privileges." – Dennis Williamson Apr 15 '10 at 13:57
This should work. it creates an array of all drives connected that can be accessed from a for loop. you can do what ever you want with the drives now. i have provided two examples to show how it works.
@echo off
setlocal enableDelayedExpansion
cls
REM getting the output from fsutil fsinfo drives and putting it in the ogdrives variable
FOR /F "tokens=* USEBACKQ" %%F IN (`fsutil fsinfo drives`) DO (
SET ogdrives=%%F
)
REM making the drives variable the same as the ogdrives variable so it can be manipulated
set drives=!ogdrives!
REM formating the out so that it looks like 1+1+1+... for every drive that is connected
set drives=!drives:Drives^: =!
set drives=!drives:^:\=1!
set drives=!drives: =+!
REM still formating to find out how many drives there are, this bit gets rid of any letters there are
set charms=0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ
for /L %%N in (10 1 62) do (
for /F %%C in ("!charms:~%%N,1!") do (
set drives=!drives:%%C=!
)
)
REM last for finding out the number, this removes the last characters since it is a leading + that shouldnt be there
set drives=!drives:~0,-1!
REM num is now the variable that contains the number of drives connected
set /a num=!drives!
REM reseting the drives variable to the original output so it can be manipulated again
set drives=!ogdrives!
REM this time it is being formated to list the drives as a solid string of drive letters like ABCD
set drives=!drives:Drives^: =!
set drives=!drives:^:\=!
set drives=!drives: =!
REM this is to iterate through that string of drive letters to seperate it into multiple single letter variables that are correlated to a number so they can be used later
:loop
REM the iter variable holds how many times this has looped so that when it hits the final drive it can exit
set /a iter=!iter!+1
REM the pos variable is the position in the string of drive letters that needs to be taken out for this iteration
set /a pos=!iter!-1
REM this sets the driveX variable where X is the drives correlated number to the letter of that drive from the long string of drive letters by using the pos variable
set drive!iter!=!drives:~%pos%,1!
REM this is checking to see if all drives have been assigned a number and if it has it will exit the loop
if !iter!==!num! goto oloop
goto loop
:oloop
REM drives are stored in variables %driveX% where X represents the drive number
REM the number of drives are stored in the %num% variable
REM below is an example for iterating through drives
REM this is an example of how to use the information gathered to iterate through the drives
REM we are using a for loop from 1 to the number of drives connected
for /L %%n in (1 1 !num!) do (
REM for every drive that is connected this will be ran
REM %%n contains a number which will increase since its a for loop
REM the drive driveX variable can then be used since drive1=A and drive2=B etc
echo drive %%n is !drive%%n!
REM you can see how i have embedded a variable inside a variable to create an array of sorts.
)
pause
exit
REM the actual variable names are drive1 drive2 drive3 but so that we can iterate through them we can just use a for loop and put the number in the variable
REM one way you can use this is with the where command since it will only search one drive at a time
REM you can do this like this
for /L %%n in (1 1 !num!) do (
where /R !drive%%n!:\ *.txt
)
REM this will return a list of all txt files in the entire system since it searches all drives.
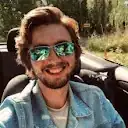
- 11
- 4
-
Pierre.Vriens , this uses the 'fsutil fsinfo drives` command to get a list of all drives connected to the computer as their drive letter, then it formats the output to change itself into a math equations that makes it so that for every drive letter it adds one so that it knows how many drives there are. Then it formats the output again to make a string that contains just the drive letters IE "ACDE" if there are four drives connected that are A:\ C:\ D:\ and E:\ then it iterates through that string and makes a variable for every drive letter as !drive%%n! which can be used from a for loop. – John Gibbons Sep 03 '18 at 04:21
It's going to be much easier, and far more useful to go with VBScript in that case. http://authors.aspalliance.com/brettb/VBScriptDrivesCollection.asp

- 2,055
- 14
- 12
-
VBScript is a dead language, I wouldn't recommend anybody learn it if they don't know it already. PowerShell does everything VBScript does, plus more, it's widely supported, and more natural to use (CLI). – Chris S Apr 18 '10 at 13:27
You can coax
wmic volume get driveletter
to be your data source here. Iterating can then be done with standard for /f
.

- 1,823
- 11
- 13
You can try:
for %%i in (C D E F G H I J K L M N O P Q R S T U V W X Y Z) DO @if exist %%i: @echo %%i:
The main limitation with this code is the existance of a CD/DVD drive with no disk. It causes a carp for the user to insert a disk. If you have all CD/DVD drives mapped to Z: you could avoid the carp by removing the final Z in the set.
Rob

- 2,766
- 1
- 17
- 22
This works for me from msys, you didn't specify which bash interpreter you are using (the main ones would be msys or cygwin).
for i in `mount|grep "^.: "|cut -c1`; do
echo iterating over drive $i
done

- 1,729
- 3
- 14
- 24
-
-
1Oh my bad, I misread 'batch' as 'bash'. Well, I'll leave this post here in case someone wants to iterate in bash in windows in the future. – davr Apr 15 '10 at 22:07
-
+1 for "I'll leave this post here in case someone wants to iterate in bash in windows in the future" – mahmoud nezar sarhan Jun 28 '17 at 06:19