hi i'am not really fluent in bash shell script, i'am doing some compilation using gcc that executed by bash script, how do I know (check) if the compilation was successful or not? any example?
4 Answers
Since you are doing this in a script, you could alternatively check the exit code of the commands as you run with the $?
variable.
You could do something like:
./configure
if [ $? -ne 0 ]
then
echo Configure failed
exit 1
fi
make
if [ $? -ne 0 ]
then
echo make failed
exit 1
fi
make install
if [ $? -ne 0 ]
then
echo make install failed
exit 1
fi
echo All steps Succeeded
Personally I tend to be more verbose and use longer forms in scripts because you never know who will be maintaining it in the future.
If it was a one off command line run i would use the method that Dennis and mibus have already mentioned

- 36,995
- 5
- 52
- 95
gcc -o foo foo.c && echo OK
:)

- 816
- 4
- 5
-
i don't get access directly to that command, it's the usual compilation scheme, like `./configure blah blah` then `make` and `make install` i need to know the result from each that command whether compilation has an error or not – uray Mar 26 '10 at 22:00
-
I'm not clear what you mean by "i don't get access directly to that command". Are you saying these commands are within another shell script? The '&&' is usually the best method when using configure, make, make install. If there is an error, then the command should print the error and then stop, and you will see the error as the last thing printed to STDERR (Which is presumably printed to your screen or a logfile). Many people use semicolons like 'configure ; make ; make install', but this is bad because any error could get lost in the flood of output. – Stefan Lasiewski Mar 26 '10 at 23:11
-
So it'd be: #!/bin/bash ./configure || (echo configure failed; exit 1) make || (echo make failed; exit 1) make install || (echo make install failed; exit 1) – mibus Mar 31 '10 at 04:04
-
Er, it swallowed my newlines. Insert them after "/bin/bash" and each ")"... – mibus Mar 31 '10 at 04:05
Your question stated "compilation using gcc", but I see in your comment that you're actually using configure
and make
. That should have been specified in your question.
You can use the same technique that mibus showed.
./configure foo && make && make install && echo OK
This will not proceed to the next step unless the previous one completed successfully and if all goes well it will print "OK".

- 60,515
- 14
- 113
- 148
A cautionary note is that WARNINGs which may be significant to the final binary generation are not treated as errors. So in any event, I would not totally trust the exit code as to whether your compilation/linking is correct. You still should verify the output.
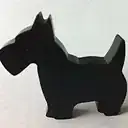
- 11,698
- 28
- 51
- 65