Javascript (using external library) (235 bytes)
Jeez this was hard! Well...my library wasn't really the right task for this haha. But I liked the challenge
(x,y)=>{r=x-1;s=y-1;m=Math.max(r,s);n=Math.min(r,s);l=_.RangeDown(s,y).WriteLine(z=>_.Range(0,x).Write("",w=>z==0&&w==0?"X":(z==w||(z==s&&w>=n)||(w==r&&z>=n))?"#":"."));return l+"\r\nMove count: "+(l.length-l.split("#").join("").length)}
Link to lib: https://github.com/mvegh1/Enumerable
Code explanation: Create function of 2 variables. Store x-1 and y-1 into variables. Store max and min of those into variables. Create a vertically descending range of numbers from (y-1) for a count of y. For each element on the vertical range, write a line for the current element, according to the complex predicate. That predicate creates an ascending range of integers from 0, for a count of x. For each element in that range, concatenate into 1 string according to a complex predicate. That predicate checks if on bottom left, else checks if on diagonal, else checks we're at X or Y border. Finally, all of that was stored in a variable. Then to get the move count, we basically just count the #'s. Then concatenate that to the stored variable, and return the result
That was a mouthful haha. The screenshot has the wrong bytecount because I found a way to save 4 bytes while posting this
EDIT: I see other answers aren't putting "Move count: " in their output, but mine is. If that isn't a requirement, that shaves a bunch of bytes...
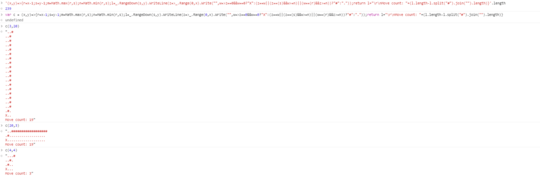
7It isn't, the solutions will require LOTS of editing – Blue – 2016-04-03T12:21:52.423
4This challenge, like the suggested duplicate, is a trivially simple problem that is non-trivial to golf, which is a great combination. Despite the similarity, this challenge requires a different approach and well golfed solutions to the previous challenge cannot be trivially amended to be competitive here. – trichoplax – 2016-04-03T12:30:04.110
It could do with a more distinguishing title though... – trichoplax – 2016-04-03T12:33:57.147
yeah, any ideas? – ericw31415 – 2016-04-03T12:42:15.630
@CoolestVeto This will actually be a great deal harder, since in that one, diagonal movements are not allowed. – ericw31415 – 2016-04-03T12:43:27.370
Close-voters, think why you want to close it. Then justify it. Then try to counter your justification. If you still think it should be closed, comment with your justification. If the justification doesn't hold up, please retract your vote. If it does hold up, please vote to reopen when it is fixed. – wizzwizz4 – 2016-04-03T12:45:04.023
By the way, that other question was also created by me. I based this one off of that one, but the answers will most likely be totally different. – ericw31415 – 2016-04-03T12:50:43.313
I think this is a great challenge. IMO it's much harder than the last one. – James – 2016-04-03T15:15:43.110
@ericw31415 What are the minimum values of the two input numbers? – Luis Mendo – 2016-04-03T15:32:24.117
1@LuisMendo The minimum is the smallest possible grid; 1 by 1. – ericw31415 – 2016-04-03T16:01:00.280