Commodore VIC-20/C64/128 and TheC64Mini, 101 tokenized BASIC bytes
Here is the obfuscated listing using Commodore BASIC keyword abbreviations:
0dEfnb(x)=sG(xaNb):inputa$:fOi=1tolen(a$):b=64:c$=mI(a$,i,1):fOj=0to6
1?rI(str$(fnb(aS(c$))),1);:b=b/2:nEj:?" ";:nE
Here for explanation purposes is the non-obfuscated symbolic listing:
0 def fn b(x)=sgn(x and b)
1 input a$
2 for i=1 to len(a$)
3 let b=64
4 let c$=mid$(a$,i,1)
5 for j=0 to 6
6 print right$(str$(fn b(asc(c$))),1);
7 let b=b/2
8 next j
9 print " ";
10 next i
The function fn b
declared on line zero accepts a numeric parameter of x
which is AND
ed with the value of b
; SGN is then used to convert x and b
to 1
or 0
.
Line one accept a string input to the variable a$
, and the loop starts (denoted with i
) to the length of that input. b
represents each bit from the 6th to 0th bit. c$
takes each character of the string at position i
.
line 5 starts the loop to test each bit position; right$
is used in line 6 to remove a auto-formatting issue when Commodore BASIC displays a number, converting the output of fn b
to a string; asc(c$)
converts the current character to its ascii code as a decimal value.
Line 7 represents the next bit value. The loop j
is ended before printing a space, then the last loop i
is ended.
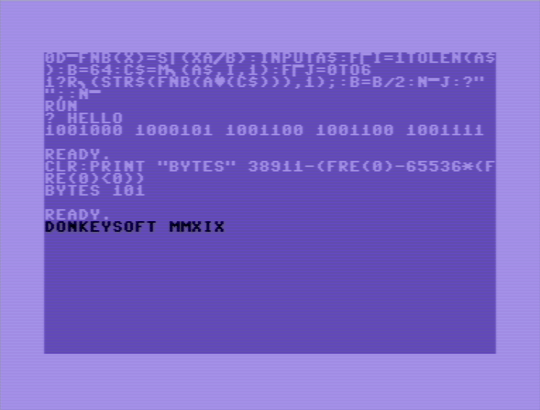
1
We had the reversed asked: http://codegolf.stackexchange.com/questions/35096/convert-a-string-of-binary-characters-to-the-ascii-equivalents
– hmatt1 – 2015-01-23T00:57:12.7871I noticed that. There's an anecdote for why I asked this question. I encouraged my friend to learn programming, and he took a java class last summer where each student had to pick a project. He told me he wanted to translate text to binary, which I then did (to his dismay) in python 3 in 1 line (a very long line). I find it incredible that his project idea can be distilled down to 8 bytes. – ericmarkmartin – 2015-01-23T04:12:50.380
1that's cool, thanks for sharing! I do like easier questions like this because it gives more people a chance to participate and generates lots of content in the form of answers. – hmatt1 – 2015-01-23T04:22:50.713
Can I take input via a file (as specified at http://meta.codegolf.stackexchange.com/a/2452/30076)?
– bmarks – 2015-01-23T16:08:47.367I would prefer that you take a string as input, but if you feel that you can provide an interesting and/or unique solution by using a file for input, please feel free to do so. – ericmarkmartin – 2015-01-27T18:05:47.393
Are the spaces necessary? – idrougge – 2017-11-02T14:25:12.730
1Does it has to be ASCII? i.e., if a technology is not ASCII compatible, could the results reflect that? – Shaun Bebbers – 2019-04-13T11:13:27.970
1Can we assume ASCII printable 32-127? If so, can binary strings be 7 chars with left zero-padding? – 640KB – 2019-04-15T15:29:07.670
1Is it acceptable to output with a separator other than spaces (e.g. a newline)? – caird coinheringaahing – 2019-09-03T09:51:43.047