Mathematica, no modulo!
n = 14627;
length = Ceiling[Log[10, n]];
img = Rasterize[n, RasterSize -> 400, ImageSize -> 400];
box = Rasterize[n, "BoundingBox", RasterSize -> 400, ImageSize -> 400];
width = box[[1]]; height = box[[3]];
ToExpression[
TextRecognize[
ImageAssemble[
ImageTake[img, {1, height}, #] & /@
NestList[# - width/length &, {width - width/length, width},
length - 1]]]]
Let's break it down.
First we use some "creative arithmetics" to find out how many digits are in the number: length = Ceiling[Log[10, n]];
Next, we Rasterize the number to a nice large image:
Now we query for the bounding box of that image, and populate the width and height (actually using the baseline offset instead of the image height, because MM adds some whitespace below the baseline in the image).
Next, NestList recursively subtracts the width of the image divided by the length of the string to enable ImageTake to pluck characters from the end of the image one by one, and those are reassembled by ImageAssemble to this image:
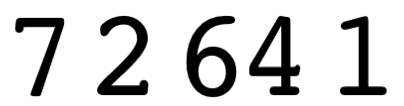
Then we pass that on to the TextRecognize function for optical character recognition, which at this image size and rasterization quality is able to impeccably recognize the final output and give us the integer:
72641
Logarithms and OCR - It's like chocolate and peanut butter!
New and improved
This version pads out the number to deal with the obstinate behavior of TextRecognize with small numbers, and then subtracts out the pad at the end. This even works for single-digit numbers!
Though, why you would run a reverse routine on a single number is a mystery to me. But just for the sake of completeness, I even made it work for inputs of zero and one, which would normally break because the floored log doesn't return 1 for them.
n = 1;
pad = 94949;
length = If[n == 1 || n == 0, 1, Ceiling[Log[10, n]]];
img = Rasterize[n + (pad*10^length), RasterSize -> 400,
ImageSize -> 400];
padlength = length + 5;
box = ImageDimensions[img];
width = box[[1]]; height = box[[2]];
reversed =
ImageResize[
ImageAssemble[
ImageTake[img, {1, height}, #] & /@
NestList[# - width/padlength &, {width + 1 - width/padlength,
width}, padlength - 1]], 200];
recognized = ToExpression[TextRecognize[reversed]];
(recognized - pad)/10^5
2I'm voting to close this as off-topic because this lacks an objective validity criterion - "be creative" is subjective. – Mego – 2016-04-22T07:58:14.830
@Mego But two people voted to keep it open, and I don't understand why
– cat – 2016-04-22T14:28:28.457@Mego Man, the popularity-contest tag itself uses the word "creativity" as criteria. I don't know how 2 years later this question could be decided to be off topic. – duci9y – 2016-04-23T04:14:19.137
@duci9y We have since decided, as a community, that popcons must have objective validity criteria for what qualifies as a valid answer, and what specific qualities make an answer better than another. Relevant meta post: http://meta.codegolf.stackexchange.com/a/8093/45941
– Mego – 2016-04-23T04:16:11.843Nope, uint all the way. – duci9y – 2014-03-03T17:35:42.227
Unicode can be embedded in other contexts than just HTML. That RTL override codepoint could appear anywhere – Gareth – 2014-03-03T17:35:46.363
@Gareth I updated the question. – duci9y – 2014-03-03T17:37:59.640
1
Is it a duplicate of http://codegolf.stackexchange.com/questions/2823/shortest-way-to-reverse-a-number ?
– microbian – 2014-03-03T18:02:28.2573@microbian No, that one was code-golf. This one is popularity-contest. – Victor Stafusa – 2014-03-03T18:03:21.527
You probably need more exclusions if you want to see genuine creativity. e.g. Database tables, lists in languages where a list functions like an array. – Jonathan Van Matre – 2014-03-03T18:14:56.053
Can we use python generator objects? – Jayanth Koushik – 2014-03-03T18:20:55.147
By "cannot use strings", do you mean that there cannot be any strings, or that we cannot use strings to reverse the integer? – Justin – 2014-03-03T18:24:16.007
You can use generators @JayanthKoushik. No strings at all. – duci9y – 2014-03-03T18:26:05.400
@Gareth Your comment freaked me out a little. "Did I really post something an hour ago??" :-) – Gareth – 2014-03-03T18:26:07.133
@Gareth How is this possible? I am so confused... – Justin – 2014-03-03T18:28:14.220
@JonathanVanMatre What do you suggest to open it up more? Should I forbid the use of modulus, or something like that? – duci9y – 2014-03-03T18:28:38.377
2
People will be ticked if you start changing rules now. It seems to be going fine to me, just run your next challenge through the sandbox first: http://meta.codegolf.stackexchange.com/questions/1117/proposed-questions-sandbox-mark-ix
– None – 2014-03-03T18:29:42.5102What if
1230
is the input? Are we allowed to output321
? (Otherwise, Strings are necessary). – Justin – 2014-03-03T18:34:39.883@Quincunx not really, see my answer – user12205 – 2014-03-03T18:41:28.383
The question is now ambiguous? What do you mean by 'suggest not using modulo'? Can we use it or can we not? Be clear. Also how can the rule be changed all of a sudden where most of the answers so far are using that modulo. By prohibiting modulo solutions will try to use arrays indirectly, like through lists and similar. Is that allowed? – microbian – 2014-03-03T18:52:43.350
The leading zeros example doesn't make much sense since it doesn't reverse. – Howard – 2014-03-03T18:52:44.207
@Howard Sorry :( updated. – duci9y – 2014-03-03T18:53:39.120
@microbian It is suggested not to use modulo, because that is the obvious solution, and this is a popularity contest. – duci9y – 2014-03-03T18:54:16.450
and everyone uses modulo! – TheDoctor – 2014-03-03T21:27:13.497