8088 Assembly, IBM PC DOS, 164 159 156 155 bytes
Binary:
00000000: d1ee 8a0c 03f1 53fd ac3a d075 0343 e2f7 ......S..:.u.C..
00000010: 85db 741c 5f8a d043 f6c3 0174 0a57 bd64 ..t._..C...t.W.d
00000020: 0155 83eb 0374 0957 bd5d 0155 4b4b 75f7 .U...t.W.].UKKu.
00000030: 8ad0 2c2f 7213 518a f0b0 24b1 31bf 6a01 ..,/r.Q...$.1.j.
00000040: fcf2 aefe ce75 fa59 57e2 bc5a 85d2 740c .....u.YW..Z..t.
00000050: b409 cd21 b220 b402 cd21 ebef c364 6f75 ...!. ...!...dou
00000060: 626c 6524 7472 6970 6c65 246f 6824 6f6e ble$triple$oh$on
00000070: 6524 7477 6f24 7468 7265 6524 666f 7572 e$two$three$four
00000080: 2466 6976 6524 7369 7824 7365 7665 6e24 $five$six$seven$
00000090: 6569 6768 7424 6e69 6e65 24 eight$nine$
Build and test executable using xxd -r
from above, or download PHONE.COM.
Unassembled listing:
D1 EE SHR SI, 1 ; point SI to DOS PSP (80H) for input string
8A 0C MOV CL, BYTE PTR[SI] ; load input string length into CX
03 F1 ADD SI, CX ; move SI to end of input
53 PUSH BX ; push a 0 to signal end of output stack
CHAR_LOOP:
FD STD ; set LODS direction to reverse
AC LODSB ; load next char from [SI] into AL, advance SI
3A D0 CMP DL, AL ; is it same as previous char?
75 03 JNZ NEW_CHAR ; if not, it's a different char
43 INC BX ; otherwise it's a run, so increment run length
E2 F7 LOOP CHAR_LOOP ; move on to next char
NEW_CHAR:
85 DB TEST BX, BX ; is there a run greater than 0?
74 1C JZ GET_WORD ; if not, look up digit name
5F POP DI ; get name for the current digit
8A D0 MOV DL, AL ; save current char in DL
43 INC BX ; adjust run count (BX=1 means run of 2, etc)
F6 C3 01 TEST BL, 1 ; is odd? if so, it's a triple
74 0A JZ IS_DBL ; is even, so is a double
57 PUSH DI ; push number string ("one", etc) to stack
BD 0164 MOV BP, OFFSET T ; load "triple" string
55 PUSH BP ; push to stack
83 EB 03 SUB BX, 3 ; decrement run count by 3
74 09 JZ GET_WORD ; if end of run, move to next input char
IS_DBL:
57 PUSH DI ; push number string to stack
BD 015D MOV BP, OFFSET D ; load "double" string
55 PUSH BP ; push to stack
4B DEC BX ; decrement by 2
4B DEC BX
75 F7 JNZ IS_DBL ; if not end of run, loop double again
GET_WORD:
8A D0 MOV DL, AL ; save current char into DL
2C 2F SUB AL, '0'-1 ; convert ASCII char to 1-based index
72 13 JB NOT_FOUND ; if not a valid char, move to next
51 PUSH CX ; save outer loop counter
8A F0 MOV DH, AL ; DH is the index to find, use as scan loop counter
B0 24 MOV AL, '$' ; word string is $ delimited
B1 31 MOV CL, 031H ; search through length of word data (49 bytes)
BF 016A MOV DI, OFFSET W ; reset word data pointer to beginning
FC CLD ; set DF to scan forward for SCAS
SCAN_LOOP:
F2/ AE REPNZ SCASB ; search until delimiter '$' is found in [DI]
FE CE DEC DH ; delimiter found, decrement counter
75 FA JNZ SCAN_LOOP ; if counter reached 0, index has been found
59 POP CX ; restore outer loop position
57 PUSH DI ; push string on stack
NOT_FOUND:
E2 BC LOOP CHAR_LOOP ; move to next char in input
OUTPUT_STACK:
5A POP DX ; get string from top of stack
85 D2 TEST DX, DX ; it is the last?
74 0C JZ EXIT ; if so, exit
B4 09 MOV AH, 09H ; DOS display string function
CD 21 INT 21H ; write string to console
B2 20 MOV DL, ' ' ; load space delimiter
B4 02 MOV AH, 02H ; DOS display char function
CD 21 INT 21H ; write char to console
EB EF JMP OUTPUT_STACK ; continue looping
EXIT:
C3 RET ; return to DOS
D DB "double$"
T DB "triple"
W DB "$oh$","one$","two$","three$","four$","five$","six$","seven$","eight$","nine$"
TL;DR:
The input string is read right to left to make it easier to find a triple. The output is pushed onto the x86 stack to simplify reversing out the display order and also facilitate rearranging the "double" and "triple" words to precede the digit's name.
If the next digit is different than the last, the name is looked up in the list of words and pushed on to the stack. Since there's no formal concept of an "indexed array of variable-length strings" in machine code, the list of words is scanned i
(the word's index) number of times for the string delimiter ($
) to find the corresponding word. Helpfully, x86 does have a pair of short instructions (REPNZ SCASB
which is similar to memchr()
in C), that simplifies this (thanks CISC!).
If the digit is the same as the previous one, the counter for the length of a "run" is incremented and continues looping leftward on the input. Once the run is over, the digit's name is taken from the stack since it will need to placed after the "double" or "triple" for each grouping. If the length of the run is odd (and run length is > 1
), the digit's name followed by the string "triple" is pushed to the stack and the run length is reduced by 3. Since the run length will now be even, the step is repeated for "double" until the run length is 0.
When the input string has reached the end, the stack is dumped out with each saved string written to the screen in reverse order.
I/O:
A standalone PC DOS executable, input from command line output to console.
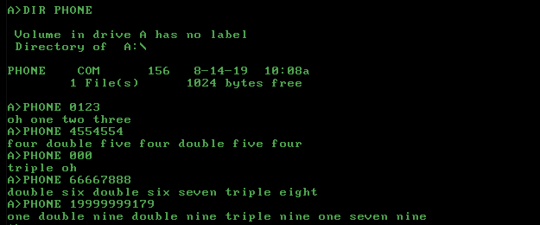
Download and test PHONE.COM.
38Anyone interested in "speech golf" should note that "double six" takes longer to say than "six six". Of all the numerical possibilities here, only "triple seven" saves syllables. – Purple P – 2019-08-13T04:40:19.303
13@Purple P: And as I'm sure you know, 'double-u double-u double-u'>'world wide web'.. – Chas Brown – 2019-08-13T04:43:16.477
11I vote to change that letter to "dub". – Hand-E-Food – 2019-08-13T04:45:11.957
2You should have used non-English/standard digit names for all digits to avoid build-ins… – Adám – 2019-08-13T06:12:19.963
"oh" and not "zero"? – Shaun Bebbers – 2019-08-13T10:20:38.287
So
oh
andzero
are both acceptable? – Shaun Bebbers – 2019-08-13T12:01:09.587@ShaunBebbers Is there a builtin in your language? ;) – V. Courtois – 2019-08-13T12:29:30.130
@recursive the test cases show
9999999
should bedouble nine double nine triple nine
, notdouble nine double nine double nine nine
as your comment suggests is correct? – 640KB – 2019-08-13T17:33:06.903I think I misread the spec. It says "When the same digit is repeated four or more times, write "double number" for the first two digits and evaluate the rest of the string." However, I read it as "first two pairs of digits". @640KB: I think you're totally correct. – recursive – 2019-08-13T17:53:33.987
8I know this is only an intellectual exercise, but I have in front of me a gas bill with the number 0800 048 1000, and I would read that as "oh eight hundred oh four eight one thousand". The grouping of digits is significant to human readers, and some patterns such as "0800" are treated specially. – Michael Kay – 2019-08-14T08:29:33.087
3@PurpleP Anyone interested in clarity of speech, however, especially when speaking over the phone, might want to use "double 6" since it's clearer that the speaker means two sixes and didn't repeat the number 6 accidentally. People aren't robots :P – user91988 – 2019-08-14T20:47:43.020