Python, 456 429 381
import turtle as t
L="fl"
R="fr"
d=L*3+R*3
b=(d+R)*3
a=[b,120,L*3+"fflflffflflfrflflfffl"+R*4+"flf",90,b+"ffrfrflffrffrfrfrflflf",120,(R*5+L*5+R+L)*5+"rrfr"+L*5+R*2+L*2+R*4+"f",72,(d+"f")*5+"rfl"+((d+"b")*5)[:-1],120]
l=t.lt
f=t.fd
b=t.bk
r=t.rt
p=input()*2-2
t.setup(.9,.9)
t.goto(-200,150)
t.clear()
for c in a[p]:exec c+"(a[p+1])"
t.getscreen().getcanvas().postscript(file="o")
I implemented a primitive interpreter with l r f b
as operators that move the turtle cursor around at the angle of the shapes. At one time, it turns only one angle. I compressed the strings by reusing strings (kind of like psuedo-subroutines), other than that, I didn't check to see if I was using the best path. It outputs to a postscript file.
A little explanation of un-golfed code:
import turtle as t
Left="fl"
Right="fr"
diamond= Left*3 + Right*3
tetrahedron=(d+R)*3 #used to be b
Imports the built-in turtle module and defines the macros that shorten the strings. The turtle module uses commands to move a 'turtle' around the screen (ie forward(100), left(90))
netList=[
#tetrahedron
tetrahedron,120,
#cube
Left*3+"fflflffflflfrflflfffl"+Right*4+"flf",90,
#octohedron, builds off the tetrahedron
tetrahedron+"ffrfrflffrffrfrfrflflf",120,
#dodecahedron
(Right*5 + Left*5 + Right + Left)*5
+"rrfr"+
Left*5 + Right*2 + Left*2 + Right*4 + "f",72,
#icosahedron
(diamond+"f")*5 +"rfl"+((diamond+"b")*5)[:-1],120
]
This list holds the angles and movement sequences. The tetrahedron was saved to reuse with the octohedren.
l=t.left
f=t.forward
b=t.back
r=t.right
This is the part that i like, it makes single character local functions so the calls can be shortened and automated through pre-defined strings.
input=int(raw_input())*2-2
t.setup(.9,.9)
t.goto(-200,150)
t.clear()
This starts by taking the input (between 1 and 5), and converting that to an index that points to the shape string in the netList. These setup turtle to show the whole net. These could be left out if the task was just to draw them, but since we need a picture output they are needed.
for command in netList[input]:
exec command+"(netList[input+1])"
t.getscreen().getcanvas().postscript(file="o")
The for loop takes the commands in the command sequence string and executes them, so for a string like "fl", this executes "forward(angle);left(angle);" by calling the newly created local functions. the last line outputs a file called 'o' that is in postscript format format using turtle function.
To run:
Copy it into a file and run it from there. When you run it, it will wait for a number input between 1 and 5 (i just changed it so that it asks before setting up turtle). When you input a number, a window pops up and draws the net. if you want it to go faster you can add t.speed(200)
before setup
.
You can copy-paste it into the interpreter, but when raw_input()
is called it consumes the next string you input "t.setup(.9,.9)"
instead of a number. So if you do this, copy up until raw_input()
, input a number, than copy paste the rest. It is intended to be run as a whole. Or you could copy it into a function and call it.
Here are it's outputs (converted from postscript):
Note: the position of these in the window has changed, but their overall shape is the same.
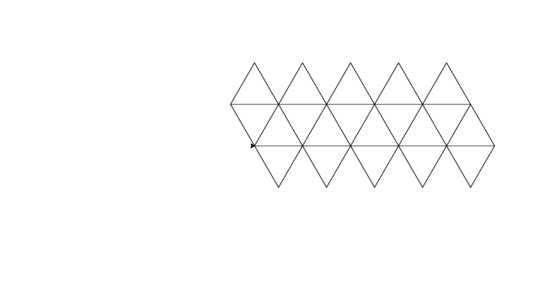
It's a little brute force for code golf, but I got tired of trying to find a consistent pattern between the shapes.
Very close. The dodecahedron is definitely more tricky. – felipa – 2013-02-12T07:54:36.283
@Raufio It's very nice. Is it not possible to define a triangle (or square or pentagon) and then just rotate/move it about? Or is that effectively what you have done? – felipa – 2013-02-12T21:52:12.370
Effectively, yes that is what I did, but with larger shapes. For instance, the icosahedron is drawn by drawing two triangles, one on top of the other, and moving forward 5 times, then resetting at a new location, drawing the diamond again moving back then repeating 5 times.
d
is the string that does the two triangles, so it is(d+'f')*5+setupPosition+(d+'b')*5
– Raufio – 2013-02-12T22:44:14.737@Raufio The golfed code doesn't work for me. It opens a window that is mostly blank. If I then press return I get p=(ord(raw_input())-49)*2
TypeError: ord() expected a character, but string of length 0 found – felipa – 2013-02-13T10:43:12.017
@Raufio I tried it line by line in ipython and I don't really get it. I mean what is t.setup(.9,.9) t.goto(-200,150) t.clear() for? And then what is p=(ord(raw_input())-49)*2 meant to do? – felipa – 2013-02-13T10:46:49.187
1@felipa
setup
makes the turtle window to be big enough to hold the net. Same thing withgoto
, it moves the 'turtle' to -200, 150.clear
clears the line made bygoto
. Their just commands for setting up drawing.p=(ord(raw_input())-49)*2
takes a number, 1 through 5, corresponding to what shape you want. – Raufio – 2013-02-13T15:53:43.240@Raufio Oh I take it back. It's beautiful :) – felipa – 2013-02-13T16:11:20.857