Java, 1552
import java.awt.*;import java.awt.image.*;import java.io.*;import javax.imageio.*;class
A{public static void main(String[]x)throws
Exception{String[]a={"33623368356:356;66","33413341334535463547354735473444","33823382338:3586338>358>358>358?88","66456:466:466845684668466766","334144453546354635474746464646464647354634463446344744","88456:466:466:4668458<468<468<468:456846684668466788","33343535353231333535313133353447353434353534313133353447353545343535313133353447353545343444","33513351335233593554335433593554335935543359355433593559355835593559355935593455","33:233:233:433:B35:833:833:B35:833:B35:833:B35:833:B35:B35:833:B35:B35:B35:B35:C::","66566:576:57696869576969586969586:586969576969586857685868586766","334155453546354635463547594658465846584658473546354634463546344635463446354634463547584657465746574657473546344634463446344755","::456:466:466:466:466845:@46:@46:@46:@46:>4568466:4668466:4668466:4668466:4668466845:>46:>46:>46:>46:<45684668466846684667::","333531333434343132333434353531313335343434323232323334343435353231333133343556343434313233323335345935353532313331313132333233353535343557343133343556343434355934353535593432333234355935323335345935323335345935323335345935343459313334353455"};BufferedImage
m=new BufferedImage(1300,1300,1);Graphics2D g=m.createGraphics();g.translate(500,500);String
s=a[Integer.valueOf(x[0])-1];int f=1,i=0,n,t;while(i<s.length()){n=s.charAt(i++)-48;t=s.charAt(i++);while(t-->48){g.drawLine(0,0,20,0);g.translate(20,0);g.rotate(f*Math.PI*2/n);}f=-f;}ImageIO.write(m,"png",new File("o.png"));}}
Ungolfed:
import java.awt.Graphics2D;
import java.awt.geom.AffineTransform;
import java.awt.image.BufferedImage;
import java.io.File;
import javax.imageio.ImageIO;
public class A {
static int f = 1;
static Graphics2D g;
static void fixtrans() {
double[] m = new double[6];
g.getTransform().getMatrix(m);
for (int i = 0; i < 6; ++i) {
if (Math.abs(m[i] - Math.round(m[i])) < 1e-5) {
m[i] = Math.round(m[i]);
}
}
g.setTransform(new AffineTransform(m));
}
static void d(String s) {
for (int i = 0; i < s.length();) {
int n = s.charAt(i++) - '0';
int t = s.charAt(i++) - '0';
for (int j = 0; j < t; ++j) {
g.drawLine(0, 0, 20, 0);
g.translate(20, 0);
g.rotate(f * Math.PI * 2 / n);
fixtrans(); // optional, straightens some lines
}
f = -f;
}
}
public static void main(String[] args) throws Exception {
String[] a = {
"33623368356:356;66",
"33413341334535463547354735473444",
"33823382338:3586338>358>358>358?88",
"66456:466:466845684668466766",
"334144453546354635474746464646464647354634463446344744",
"88456:466:466:4668458<468<468<468:456846684668466788",
"33343535353231333535313133353447353434353534313133353447353545343535313133353447353545343444",
"33513351335233593554335433593554335935543359355433593559355835593559355935593455",
"33:233:233:433:B35:833:833:B35:833:B35:833:B35:833:B35:B35:833:B35:B35:B35:B35:C::",
"66566:576:57696869576969586969586:586969576969586857685868586766",
"334155453546354635463547594658465846584658473546354634463546344635463446354634463547584657465746574657473546344634463446344755",
"::456:466:466:466:466845:@46:@46:@46:@46:>4568466:4668466:4668466:4668466:4668466845:>46:>46:>46:>46:<45684668466846684667::",
// bad "333531333434343132333434353531313335343434323232323334343435353231333133343556343434313233323335345935353532313331313132333233353535343557343133343556343434355934353531333459343434355935323335345935323335345935323335345935323335345935353455"
"333531333434343132333434353531313335343434323232323334343435353231333133343556343434313233323335345935353532313331313132333233353535343557343133343556343434355934353535593432333234355935323335345935323335345935323335345935343459313334353455"};
BufferedImage img = new BufferedImage(1300, 1300, BufferedImage.TYPE_INT_RGB);
g = img.createGraphics();
g.translate(500, 500);
d(a[Integer.parseInt(args[0]) - 1]);
String f = args[0] + ".png";
ImageIO.write(img, "png", new File(f));
}
}
Results (trimmed, negated, joined and scaled):
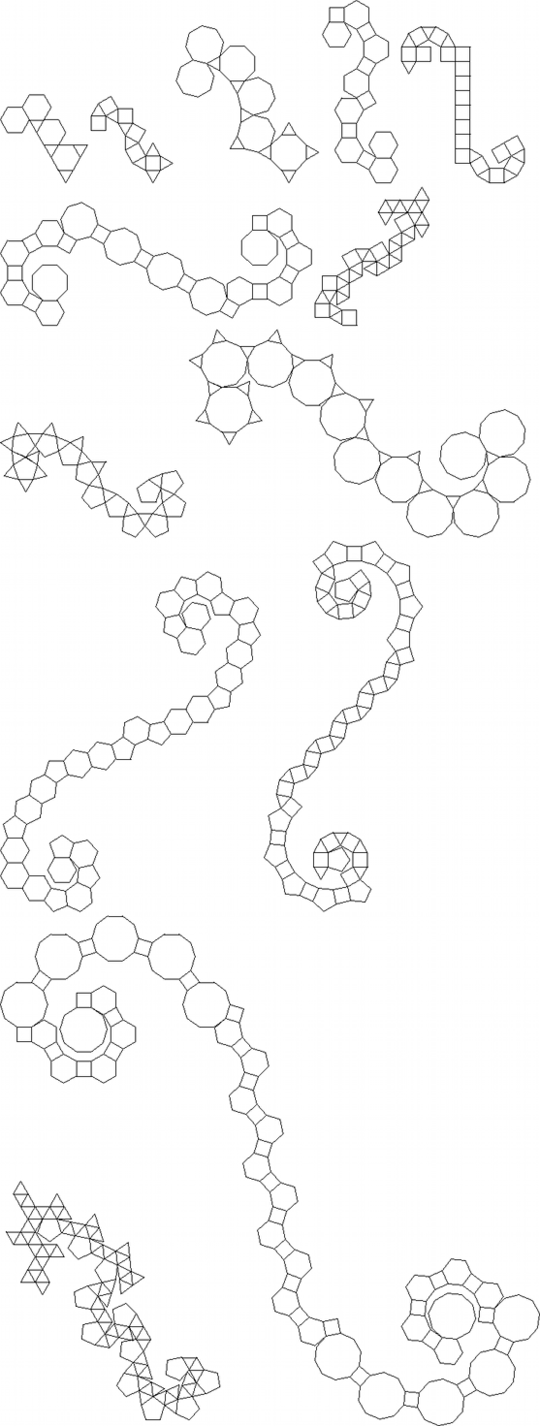
The shapes are quite unusual :) but correct as far as I can tell (let me know if you find any errors). They were generated (in a separate program) by constructing the face graph and cutting cycles in a DFS.
I'm sure this can be golfed quite a lot more using e.g. python and turtle.
Edit: oops, the last case was self-intersecting a bit. I fixed the code (by hand), here's the updated image:
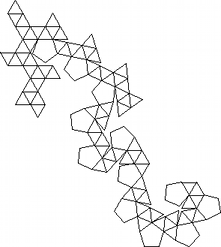
Maybe do away with the time-limit altogether? What if somebody finds this a year from now. Do you want them not to try?...Might've been better to do the Platonic first, wait, then the hard one. You may have divided the interest. For me personally (all of this is extrapolation), when I saw two similar ones, I kind of withdrew from it, feeling I didn't have the time to really look at both and plan out how to solve both. And I wouldn't want to do it any other way....On the other hand, others here have had difficulty with Part-2 challenges. See the Minsky Register Machine ones. Maybe it's not you. – luser droog – 2013-02-18T00:15:29.487
@luserdroog Thanks. Question edited. I should maybe add that I have emailed the answer to the related question around lots of people who love it! FWIW. – felipa – 2013-02-20T16:16:32.690
I don't think it's hard to do, but to golf it requires several hours of thinking and experimenting because there are many possible nets for each polyhedron and they won't compress equally well. – Peter Taylor – 2013-03-02T12:58:11.213