My attempt for redemption of my earlier faux pas ....
I liked the idea, so today I wrote a plugin for VIM to show a scrollbar 'thumb' using the signs feature of vim.
It's still VERY beta, but it's usable right now, I still have work to do on it, including typing up all the docs and comments and stuff.
I'll post the source here, but you're welcome to pull it from my Hg Repo. (Don't laugh too hard over the other stuff)
Remember... VERY beta, considering I've never written a plugin before, only dabbling in VimL over the years. (less than 12 hours from concept to working prototype! yay!)
I'll keep working on it, kinda neat. The colors are garish for a reason, easy to see what changes. It has a big bug right now, you can't make the signs all go away by toggling it off. I know how to implement this, just wanted to share.
Pictures are useful:
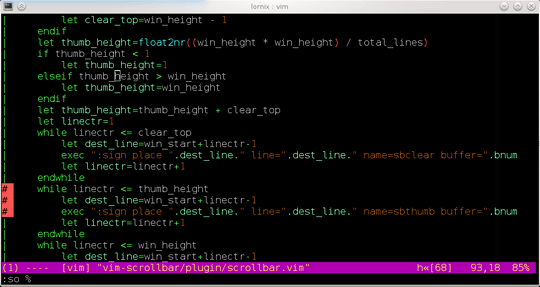
VIM Curses Scrollbar - v0.1 - L Nix - lornix@lornix.com Hg Repo
" Vim global plugin to display a curses scrollbar
" Version: 0.1.1
" Last Change: 2012 Jul 06
" Author: Loni Nix <lornix@lornix.com>
"
" License: TODO: Have to put something here
"
"
if exists('g:loaded_scrollbar')
finish
endif
let g:loaded_scrollbar=1
"
" save cpoptions
let s:save_cpoptions=&cpoptions
set cpoptions&vim
"
" some global constants
if !exists('g:scrollbar_thumb')
let g:scrollbar_thumb='#'
endif
if !exists('g:scrollbar_clear')
let g:scrollbar_clear='|'
endif
"
"our highlighting scheme
highlight Scrollbar_Clear ctermfg=green ctermbg=black guifg=green guibg=black cterm=none
highlight Scrollbar_Thumb ctermfg=red ctermbg=black guifg=red guibg=black cterm=reverse
"
"the signs we're goint to use
exec "sign define sbclear text=".g:scrollbar_clear." texthl=Scrollbar_Clear"
exec "sign define sbthumb text=".g:scrollbar_thumb." texthl=Scrollbar_Thumb"
"
" set up a default mapping to toggle the scrollbar
" but only if user hasn't already done it
if !hasmapto('ToggleScrollbar')
map <silent> <unique> <leader>sb :call <sid>ToggleScrollbar()<cr>
endif
"
" start out activated or not?
if !exists('s:scrollbar_active')
let s:scrollbar_active=1
endif
"
function! <sid>ToggleScrollbar()
if s:scrollbar_active
let s:scrollbar_active=0
" clear out the autocmds
augroup Scrollbar_augroup
autocmd!
augroup END
"call <sid>ZeroSignList()
else
let s:scrollbar_active=1
call <sid>SetupScrollbar()
endif
endfunction
function! <sid>SetupScrollbar()
augroup Scrollbar_augroup
autocmd BufEnter * :call <sid>showScrollbar()
autocmd BufWinEnter * :call <sid>showScrollbar()
autocmd CursorHold * :call <sid>showScrollbar()
autocmd CursorHoldI * :call <sid>showScrollbar()
autocmd CursorMoved * :call <sid>showScrollbar()
autocmd CursorMovedI * :call <sid>showScrollbar()
autocmd FocusGained * :call <sid>showScrollbar()
autocmd VimResized * :call <sid>showScrollbar()
augroup END
call <sid>showScrollbar()
endfunction
"
function! <sid>showScrollbar()
" not active, go away
if s:scrollbar_active==0
return
endif
"
let bnum=bufnr("%")
let total_lines=line('$')
let current_line=line('.')
let win_height=winheight(0)
let win_start=line('w0')+0 "curious, this was only one had to be forced
let clear_top=float2nr((current_line * win_height) / total_lines) - 1
if clear_top < 0
let clear_top=0
elseif clear_top > (win_height - 1)
let clear_top=win_height - 1
endif
let thumb_height=float2nr((win_height * win_height) / total_lines)
if thumb_height < 1
let thumb_height=1
elseif thumb_height > win_height
let thumb_height=win_height
endif
let thumb_height=thumb_height + clear_top
let linectr=1
while linectr <= clear_top
let dest_line=win_start+linectr-1
exec ":sign place ".dest_line." line=".dest_line." name=sbclear buffer=".bnum
let linectr=linectr+1
endwhile
while linectr <= thumb_height
let dest_line=win_start+linectr-1
exec ":sign place ".dest_line." line=".dest_line." name=sbthumb buffer=".bnum
let linectr=linectr+1
endwhile
while linectr <= win_height
let dest_line=win_start+linectr-1
exec ":sign place ".dest_line." line=".dest_line." name=sbclear buffer=".bnum
let linectr=linectr+1
endwhile
endfunction
"
" fire it all up if we're 'active'
if s:scrollbar_active != 0
call <sid>SetupScrollbar()
endif
"
" restore cpoptions
let &cpoptions=s:save_cpoptions
unlet s:save_cpoptions
"
" vim: set filetype=vim fileformat=unix expandtab softtabstop=4 shiftwidth=4 tabstop=8:
Thanks! This is very close to my stated requirement, though from the doc it seems that signs can only be drawn on the left side. Good answer though, accepted! – xiaq – 2012-07-28T09:18:20.477