7 years, 8 browsers
Not exactly language release dates because css doesn't actually have releases, but browser versions (could be thought as compiler versions maybe?)
For the following browsers, this prints the browser name, version and release date and nothing else. It does print the same thing for a few later versions, (you will see chromium is one version late in the screenshots because it's hard to download old versions of chrome by version number)
I could print the version number I used but I preferred to show the first version where the used feature is introduced.
- IE6
- IE7
- IE8
- IE9 (untested, don't have an IE9 around)
- Firefox 4
- Firefox 3.6
- Chrome 18
- Chrome 21
- Modern Browsers
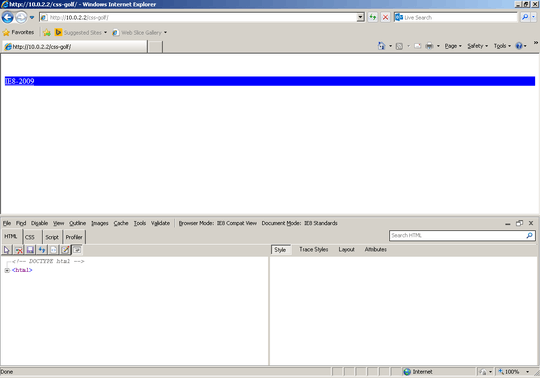
index.html
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<link rel=stylesheet href=style.css>
</head>
<body>
<div class=borderbox>
<div class="inlineblock">
IE6-2001
</div>
<div class="white">
<div class="outofbox">
<a href="#">IE8-2009</a>
</div>
<div class="inherit">
<a href="#">IE7-2006</a>
</div>
</div>
</div>
<div class="white">
<header class="filter-quirk filter-blur filter-blur-url">
IE9
</header>
</div>
<div class="flex white">
Modern Browsers - 2017
</div>
<div class="webkit-flex">
<div class="">
Chrome 21-2012
</div>
</div>
<div class="webkit-invert white flexdisable">
<div class="">
Chrome 18-2012
</div>
</div>
<svg style="position: absolute; top: -99999px" xmlns="http://www.w3.org/2000/svg">
</svg>
<div class="filter">
<svg xmlns="http://www.w3.org/2000/svg" version="1.1">
<g
transform="scale(8)"
aria-label="FF4"
id="text8419">
<rect
style="color:#000000;clip-rule:nonzero;display:inline;overflow:visible;visibility:visible;opacity:1;isolation:auto;mix-blend-mode:normal;color-interpolation:sRGB;color-interpolation-filters:linearRGB;solid-color:#000000;solid-opacity:1;fill:#ffffff;fill-opacity:1;fill-rule:nonzero;stroke:none;stroke-width:0.79374999;stroke-miterlimit:4;stroke-dasharray:none;stroke-dashoffset:0;stroke-opacity:1;marker:none;color-rendering:auto;image-rendering:auto;shape-rendering:auto;text-rendering:auto;enable-background:accumulate"
id="rect21965"
width="17.005648"
height="3.9855044"
x="-0.16825682"
y="-0.025296567" />
<path
d="m 1.0052634,1.5511362 v 0.7639843 h 0.4564063 q 0.092604,0 0.092604,0.066146 0,0.069453 -0.092604,0.069453 H 0.69107072 q -0.089297,0 -0.089297,-0.069453 0,-0.066146 0.089297,-0.066146 h 0.1785938 V 0.72431329 h -0.1785938 q -0.089297,0 -0.089297,-0.069453 0,-0.066146 0.089297,-0.066146 H 2.1793519 V 1.0484278 q 0,0.089297 -0.069453,0.089297 -0.066146,0 -0.066146,-0.089297 V 0.72431329 H 1.0052634 V 1.4155373 H 1.4848207 V 1.2667091 q 0,-0.089297 0.066146,-0.089297 0.069453,0 0.069453,0.089297 v 0.4332552 q 0,0.089297 -0.069453,0.089297 -0.066146,0 -0.066146,-0.089297 V 1.5511362 Z"
style="fill:#000000;stroke-width:0.79374999"
id="path8421" />
<path
d="m 2.9883464,1.5511362 v 0.7639843 h 0.4564063 q 0.092604,0 0.092604,0.066146 0,0.069453 -0.092604,0.069453 h -0.770599 q -0.089297,0 -0.089297,-0.069453 0,-0.066146 0.089297,-0.066146 H 2.8527475 V 0.72431329 H 2.6741537 q -0.089297,0 -0.089297,-0.069453 0,-0.066146 0.089297,-0.066146 H 4.1624349 V 1.0484278 q 0,0.089297 -0.069453,0.089297 -0.066146,0 -0.066146,-0.089297 V 0.72431329 H 2.9883464 V 1.4155373 H 3.4679037 V 1.2667091 q 0,-0.089297 0.066146,-0.089297 0.069453,0 0.069453,0.089297 v 0.4332552 q 0,0.089297 -0.069453,0.089297 -0.066146,0 -0.066146,-0.089297 V 1.5511362 Z"
style="fill:#000000;stroke-width:0.79374999"
id="path8423" />
<path
d="M 5.6692683,1.8917872 H 4.7729923 V 1.7363445 l 0.754062,-1.28322911 h 0.277813 V 1.7561883 h 0.112448 q 0.0893,0 0.0893,0.069453 0,0.066146 -0.0893,0.066146 h -0.112448 v 0.4233333 h 0.112448 q 0.0893,0 0.0893,0.066146 0,0.069453 -0.0893,0.069453 h -0.499402 q -0.0893,0 -0.0893,-0.069453 0,-0.066146 0.0893,-0.066146 h 0.251355 z m 0,-0.1355989 V 0.58871439 h -0.07938 L 4.9019713,1.7561883 Z"
style="fill:#000000;stroke-width:0.79374999"
id="path8425" />
<path
d="M 8.2881171,1.6077068 H 6.9585859 q -0.089297,0 -0.089297,-0.069453 0,-0.066146 0.089297,-0.066146 h 1.3295312 q 0.089297,0 0.089297,0.066146 0,0.069453 -0.089297,0.069453 z"
style="stroke-width:0.79374999"
id="path8422" />
<path
d="m 8.9582054,0.90656104 q 0,-0.14882812 0.1785937,-0.31749999 0.1819011,-0.17197916 0.4365625,-0.17197916 0.2414323,0 0.4233333,0.17197916 0.1852081,0.17197916 0.1852081,0.39687498 0,0.14882817 -0.0893,0.28111977 Q 10.006617,1.3960402 9.7056533,1.67716 L 9.0210439,2.3154672 v 0.00992 H 10.059533 V 2.2063266 q 0,-0.089297 0.06945,-0.089297 0.06614,0 0.06614,0.089297 V 2.460988 H 8.8920596 V 2.2625506 L 9.6725804,1.5283318 q 0.2315104,-0.2282031 0.3009635,-0.3307291 0.07276,-0.102526 0.07276,-0.21497396 0,-0.17197916 -0.1422132,-0.30096353 -0.1422136,-0.12898437 -0.3307292,-0.12898437 -0.1686718,0 -0.3075781,0.0992188 -0.1355989,0.0992188 -0.1752864,0.24804686 -0.019844,0.0661458 -0.069453,0.0661458 -0.023151,0 -0.042995,-0.0165365 -0.019844,-0.0198437 -0.019844,-0.0429948 z"
style="stroke-width:0.79374999"
id="path8424" />
<path
d="m 12.207981,1.3001287 v 0.3307292 q 0,0.3902604 -0.171979,0.6349999 -0.171979,0.2447396 -0.446484,0.2447396 -0.274506,0 -0.446485,-0.2447396 Q 10.971054,2.0211183 10.971054,1.6308579 V 1.3001287 q 0,-0.39356766 0.171979,-0.63830724 0.171979,-0.24473957 0.446485,-0.24473957 0.274505,0 0.446484,0.24473957 0.171979,0.24473958 0.171979,0.63830724 z M 11.589518,0.55268084 q -0.224896,0 -0.353881,0.22820312 -0.128984,0.22489584 -0.128984,0.53578124 v 0.2943489 q 0,0.3241146 0.132292,0.5457031 0.135599,0.2182813 0.350573,0.2182813 0.224895,0 0.35388,-0.2248959 0.128984,-0.2282031 0.128984,-0.5390885 V 1.3166652 q 0,-0.32411458 -0.135599,-0.54239582 -0.132292,-0.22158854 -0.347265,-0.22158854 z"
style="stroke-width:0.79374999"
id="path8426" />
<path
d="M 13.642054,0.43692564 V 2.3253891 h 0.459713 q 0.0893,0 0.0893,0.066146 0,0.069453 -0.0893,0.069453 h -1.055026 q -0.0926,0 -0.0926,-0.069453 0,-0.066146 0.0926,-0.066146 h 0.459714 V 0.61551938 l -0.373724,0.37372394 q -0.02646,0.0264584 -0.06945,0.0264584 -0.02646,0 -0.0463,-0.0198438 -0.01654,-0.023151 -0.01654,-0.056224 0,-0.0297656 0.03638,-0.0661458 l 0.436562,-0.43656248 z"
style="stroke-width:0.79374999"
id="path8428" />
<path
d="M 15.625137,0.43692564 V 2.3253891 h 0.459713 q 0.0893,0 0.0893,0.066146 0,0.069453 -0.0893,0.069453 h -1.055026 q -0.0926,0 -0.0926,-0.069453 0,-0.066146 0.0926,-0.066146 h 0.459714 V 0.61551938 l -0.373724,0.37372394 q -0.02646,0.0264584 -0.06945,0.0264584 -0.02646,0 -0.0463,-0.0198438 -0.01654,-0.023151 -0.01654,-0.056224 0,-0.0297656 0.03638,-0.0661458 l 0.436563,-0.43656248 z"
style="stroke-width:0.79374999"
id="path8430" />
</g>
</svg>
</div>
<div class="white gradient msfilter" style=color:white>
FF3.6-2010
</div>
</body>
</html>
style.css
.borderbox {
height: 40px;
overflow: hidden;
padding-bottom: 100px;
}
.outofbox {
background: blue none repeat scroll 0 0;
margin-top: 20px;
opacity: 0;
}
.white {
color: white;
}
.outofbox a, .inherit a, .inherit a:visited, .outofbox a:visited {
color: inherit;
}
.inlineblock {
display: inline;
width: 100%;
zoom: 1;
display: inline-block;
margin-left: 100px;
text-align: right;
}
.white header{
color: black;
}
.absolute{
position: absolute;
}
.flex{
display: none;
display: flex;
}
.flex.white{
filter: invert(100%)
}
.webkit-flex{
display: none;
display: -webkit-flex;
overflow: hidden;
flex-flow: column;
height: 3em;
justify-content: flex-end;
}
.webkit-flex div{
margin-bottom: -1.1em;
}
.flexdisable{
display: -webkit-flex;
overflow: hidden;
-webkit-flex-flow: column;
-webkit-justify-content: flex-end;
height: 60px;
}
.flexdisable div{
margin-bottom: -30px;
}
.filter-quirk{
filter: url(#quirk);
}
.filter-blur{
filter: blur(100px);
-webkit-filter: blur(100px);
}
.webkit-blur{
-webkit-filter: blur(100px);
}
.webkit-invert{
-webkit-filter: invert(100%);
filter: none;
}
.filter-url-dark{
-webkit-filter: url(filter.svg#Invert);
filter: url(filter.svg#Invert) invert(100%);
}
.filter-blur-url{
filter: url(filter.svg#blur) url(filter.svg#brightness);
}
.filter{
filter: invert(100%) brightness(100%);
-webkit-filter: invert(100%) brightness(100%) blur(100px);
}
.filter svg{
position: absolute;
}
.filter svg rect{
filter: invert(100%);
-webkit-filter: invert(100%);
}
.msfilter{
/* IE 8 */
-ms-filter: "progid:DXImageTransform.Microsoft.Alpha(Opacity=0)";
/* IE 5-7 */
filter: alpha(opacity=0);
}
.opacity{
}
.black{
background: black;
}
.gradient{
width: 100px;
background: -moz-linear-gradient( 45deg, #000, #000 );
font-weight: bold;
}
filter.svg
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<link rel=stylesheet href=style.css>
</head>
<body>
<div class=borderbox>
<div class="inlineblock">
IE6-2001
</div>
<div class="white">
<div class="outofbox">
<a href="#">IE8-2009</a>
</div>
<div class="inherit">
<a href="#">IE7-2006</a>
</div>
</div>
</div>
<div class="white">
<header class="filter-quirk filter-blur filter-blur-url">
IE9
</header>
</div>
<div class="flex white">
Modern Browsers - 2017
</div>
<div class="webkit-flex">
<div class="">
Chrome 21-2012
</div>
</div>
<div class="webkit-invert white flexdisable">
<div class="">
Chrome 18-2012
</div>
</div>
<svg style="position: absolute; top: -99999px" xmlns="http://www.w3.org/2000/svg">
</svg>
<div class="filter">
<svg xmlns="http://www.w3.org/2000/svg" version="1.1">
<g
transform="scale(8)"
aria-label="FF4"
id="text8419">
<rect
style="color:#000000;clip-rule:nonzero;display:inline;overflow:visible;visibility:visible;opacity:1;isolation:auto;mix-blend-mode:normal;color-interpolation:sRGB;color-interpolation-filters:linearRGB;solid-color:#000000;solid-opacity:1;fill:#ffffff;fill-opacity:1;fill-rule:nonzero;stroke:none;stroke-width:0.79374999;stroke-miterlimit:4;stroke-dasharray:none;stroke-dashoffset:0;stroke-opacity:1;marker:none;color-rendering:auto;image-rendering:auto;shape-rendering:auto;text-rendering:auto;enable-background:accumulate"
id="rect21965"
width="17.005648"
height="3.9855044"
x="-0.16825682"
y="-0.025296567" />
<path
d="m 1.0052634,1.5511362 v 0.7639843 h 0.4564063 q 0.092604,0 0.092604,0.066146 0,0.069453 -0.092604,0.069453 H 0.69107072 q -0.089297,0 -0.089297,-0.069453 0,-0.066146 0.089297,-0.066146 h 0.1785938 V 0.72431329 h -0.1785938 q -0.089297,0 -0.089297,-0.069453 0,-0.066146 0.089297,-0.066146 H 2.1793519 V 1.0484278 q 0,0.089297 -0.069453,0.089297 -0.066146,0 -0.066146,-0.089297 V 0.72431329 H 1.0052634 V 1.4155373 H 1.4848207 V 1.2667091 q 0,-0.089297 0.066146,-0.089297 0.069453,0 0.069453,0.089297 v 0.4332552 q 0,0.089297 -0.069453,0.089297 -0.066146,0 -0.066146,-0.089297 V 1.5511362 Z"
style="fill:#000000;stroke-width:0.79374999"
id="path8421" />
<path
d="m 2.9883464,1.5511362 v 0.7639843 h 0.4564063 q 0.092604,0 0.092604,0.066146 0,0.069453 -0.092604,0.069453 h -0.770599 q -0.089297,0 -0.089297,-0.069453 0,-0.066146 0.089297,-0.066146 H 2.8527475 V 0.72431329 H 2.6741537 q -0.089297,0 -0.089297,-0.069453 0,-0.066146 0.089297,-0.066146 H 4.1624349 V 1.0484278 q 0,0.089297 -0.069453,0.089297 -0.066146,0 -0.066146,-0.089297 V 0.72431329 H 2.9883464 V 1.4155373 H 3.4679037 V 1.2667091 q 0,-0.089297 0.066146,-0.089297 0.069453,0 0.069453,0.089297 v 0.4332552 q 0,0.089297 -0.069453,0.089297 -0.066146,0 -0.066146,-0.089297 V 1.5511362 Z"
style="fill:#000000;stroke-width:0.79374999"
id="path8423" />
<path
d="M 5.6692683,1.8917872 H 4.7729923 V 1.7363445 l 0.754062,-1.28322911 h 0.277813 V 1.7561883 h 0.112448 q 0.0893,0 0.0893,0.069453 0,0.066146 -0.0893,0.066146 h -0.112448 v 0.4233333 h 0.112448 q 0.0893,0 0.0893,0.066146 0,0.069453 -0.0893,0.069453 h -0.499402 q -0.0893,0 -0.0893,-0.069453 0,-0.066146 0.0893,-0.066146 h 0.251355 z m 0,-0.1355989 V 0.58871439 h -0.07938 L 4.9019713,1.7561883 Z"
style="fill:#000000;stroke-width:0.79374999"
id="path8425" />
<path
d="M 8.2881171,1.6077068 H 6.9585859 q -0.089297,0 -0.089297,-0.069453 0,-0.066146 0.089297,-0.066146 h 1.3295312 q 0.089297,0 0.089297,0.066146 0,0.069453 -0.089297,0.069453 z"
style="stroke-width:0.79374999"
id="path8422" />
<path
d="m 8.9582054,0.90656104 q 0,-0.14882812 0.1785937,-0.31749999 0.1819011,-0.17197916 0.4365625,-0.17197916 0.2414323,0 0.4233333,0.17197916 0.1852081,0.17197916 0.1852081,0.39687498 0,0.14882817 -0.0893,0.28111977 Q 10.006617,1.3960402 9.7056533,1.67716 L 9.0210439,2.3154672 v 0.00992 H 10.059533 V 2.2063266 q 0,-0.089297 0.06945,-0.089297 0.06614,0 0.06614,0.089297 V 2.460988 H 8.8920596 V 2.2625506 L 9.6725804,1.5283318 q 0.2315104,-0.2282031 0.3009635,-0.3307291 0.07276,-0.102526 0.07276,-0.21497396 0,-0.17197916 -0.1422132,-0.30096353 -0.1422136,-0.12898437 -0.3307292,-0.12898437 -0.1686718,0 -0.3075781,0.0992188 -0.1355989,0.0992188 -0.1752864,0.24804686 -0.019844,0.0661458 -0.069453,0.0661458 -0.023151,0 -0.042995,-0.0165365 -0.019844,-0.0198437 -0.019844,-0.0429948 z"
style="stroke-width:0.79374999"
id="path8424" />
<path
d="m 12.207981,1.3001287 v 0.3307292 q 0,0.3902604 -0.171979,0.6349999 -0.171979,0.2447396 -0.446484,0.2447396 -0.274506,0 -0.446485,-0.2447396 Q 10.971054,2.0211183 10.971054,1.6308579 V 1.3001287 q 0,-0.39356766 0.171979,-0.63830724 0.171979,-0.24473957 0.446485,-0.24473957 0.274505,0 0.446484,0.24473957 0.171979,0.24473958 0.171979,0.63830724 z M 11.589518,0.55268084 q -0.224896,0 -0.353881,0.22820312 -0.128984,0.22489584 -0.128984,0.53578124 v 0.2943489 q 0,0.3241146 0.132292,0.5457031 0.135599,0.2182813 0.350573,0.2182813 0.224895,0 0.35388,-0.2248959 0.128984,-0.2282031 0.128984,-0.5390885 V 1.3166652 q 0,-0.32411458 -0.135599,-0.54239582 -0.132292,-0.22158854 -0.347265,-0.22158854 z"
style="stroke-width:0.79374999"
id="path8426" />
<path
d="M 13.642054,0.43692564 V 2.3253891 h 0.459713 q 0.0893,0 0.0893,0.066146 0,0.069453 -0.0893,0.069453 h -1.055026 q -0.0926,0 -0.0926,-0.069453 0,-0.066146 0.0926,-0.066146 h 0.459714 V 0.61551938 l -0.373724,0.37372394 q -0.02646,0.0264584 -0.06945,0.0264584 -0.02646,0 -0.0463,-0.0198438 -0.01654,-0.023151 -0.01654,-0.056224 0,-0.0297656 0.03638,-0.0661458 l 0.436562,-0.43656248 z"
style="stroke-width:0.79374999"
id="path8428" />
<path
d="M 15.625137,0.43692564 V 2.3253891 h 0.459713 q 0.0893,0 0.0893,0.066146 0,0.069453 -0.0893,0.069453 h -1.055026 q -0.0926,0 -0.0926,-0.069453 0,-0.066146 0.0926,-0.066146 h 0.459714 V 0.61551938 l -0.373724,0.37372394 q -0.02646,0.0264584 -0.06945,0.0264584 -0.02646,0 -0.0463,-0.0198438 -0.01654,-0.023151 -0.01654,-0.056224 0,-0.0297656 0.03638,-0.0661458 l 0.436563,-0.43656248 z"
style="stroke-width:0.79374999"
id="path8430" />
</g>
</svg>
</div>
<div class="white gradient msfilter" style=color:white>
FF3.6-2010
</div>
</body>
</html>
Since Pip doesn't have a Wikipedia or Esolangs page, just has a GitHub, and hasn't had any "releases" in the GitHub sense of the word, is it ineligible for use in this challenge? (It does have a version number, which is updated at every commit.)
– DLosc – 2016-09-29T03:44:01.2901@dlosc Well, I don't want to unnecessarily exclude any languages, but I want to make sure that the "release date" stays completely objective. Can you think of any better ways to enforce a release date that would include more languages? – James – 2016-09-29T04:03:39.187
4
(casually inserts Whitespace code to print 2003 in basically every single submission)
– Value Ink – 2016-09-29T05:11:50.7778"You may not use any builtins that give you information about the current version of the language you are using." My emphasis. May I look for the build date in the interpreter file? – Adám – 2016-09-29T07:49:04.487
@mego the main idea of the challenge is extremely similar, but you could not copy a single submission from the challenge and have it be competitive on this challenge. I believe this is a sufficient difference to make it not a duplicate. – James – 2016-09-29T13:08:47.750
@DJMcMayhem You could trivially modify the submissions by having them output the language's release year, and they would be competitive. – Mego – 2016-09-29T13:10:01.627
2I don't think this one is a duplicate at all. Even if it is, this challenge should be accepted as the 'original' because unlike the other one, this challenge encourages users to go out and learn about what they're using. – None – 2016-09-29T15:35:48.627
I did not see that.. I have many more reasons that this should stay open, though. – None – 2016-09-29T15:41:08.940
"Latest commit does not count, unless there is a version number somewhere in the code." How about a commit that explicitly mentions the version? (This one specifically.)
– Martin Ender – 2016-09-30T14:26:43.330If different languages require different file extensions to compile/run can we write a polyglot between the two? i.e. Does our program have to have a static name between runs? – Post Rock Garf Hunter – 2016-10-02T01:14:48.337
@wheatwizard As long as the code is the same, the file extension/name doesn't matter. – James – 2016-10-02T01:30:57.757
In some languages there are functions added to each new version (one each year). In Matlab for instance, R2012a doesn't have a
table
function since it was first introduced in R2013a. Can I use this? Note, I'm not talking about fetching the year it was introduced, but rather if it works or not in the current version... – Stewie Griffin – 2016-10-03T13:05:38.157@WeeingIfFirst That's basically what sp3000's python answer is. As long as you can show a release date and version number, that sounds fine by me. – James – 2016-10-03T14:36:57.390