11,520 generations per clock count / 10,016 x 6,796 box / 244,596 pop count
There you go... Was fun.
Well, the design is certainly not optimal. Neither from the bounding box standpoint (those 7-segment digits are huge), nor from the initial population count (there are some useless stuff, and some stuff that could certainly be made simpler), and the execution speed - well... I'm not sure.
But, hey, it's beautiful. Look:
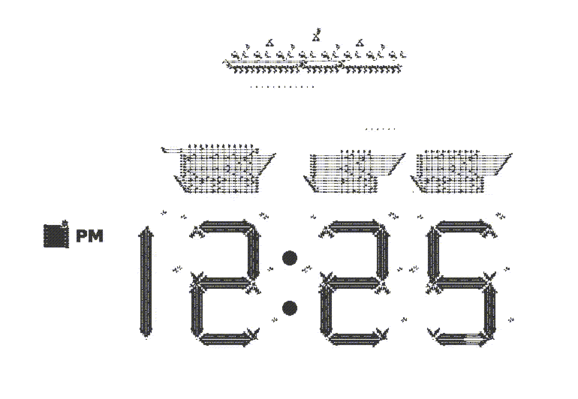
Run it!
Get the design from this gist. Copy the whole file text to the clipboard.
New: here is a version with both AM and PM indicators for the demanding.
Go to the online JavaScript Conway life simulator. Click import, paste the design text. You should see the design. Then, go to settings and set the generation step to 512, or something around those lines, or you'll have to wait forever to see the clock display updating.
Click run, wait a bit and be amazed!
Direct link to in-browser version.
Note that the only algorithm that makes this huge design useable is hashlife. But with this, you can achieve the whole clock wraparound in seconds. With other algorithms, it is impractical to even see the hour changing.
How it works
It uses p30 technology. Just basic things, gliders and lightweight spaceships. Basically, the design goes top-down:
- At the very top, there's the clock. It is a 11520 period clock. Note that you need about 10.000 generations to ensure the display is updated appropriately, but the design should still be stable with a clock of smaller period (about 5.000 or so - the clock needs to be multiple of 60).
- Then, there is the clock distribution stage. The clock glider is copied in a balanced tree, so at the end, there are 32 gliders arriving at the exact same moment to the counters stage.
- The counter stage is made using a RS latch for each state, and for each digit (we're counting in decimal). So there is 10 states for the right digit of the minutes, 6 states for the left digit of the minuts, and 12 states for the hours (both digits of the hours are merged here). For each of these groups, the counter behaves like a shift register.
- After the counting stage, there are the lookup tables. They convert the state pulses to display segments ON/OFF actions.
- Then, the display itself. The segments are simply made with multiple strings of LWSS. Each segment has it own latch to maintain its state. I could have made a simple logical-OR of the digit states to know wether a segment must be ON or OFF, and get rid of these latches, but there would be glitches for non-changing segments, when the digits are changing (because of signal delays). And there would be long streams of gliders coming from the lookup table to the digit segments. So it wouldn't be as nice-looking. And it needed to be. Yes.
Anyway, there is actually nothing extraordinary in this design. There are no amazing reactions that have been discovered in this process, and no really clever combinations that nobody thought of before. Just bits taken here and there and put together (and I'm not even sure I did it the "right" way - I was actually completely new to this). It required a lot of patience, however. Making all those gliders coming up at the right time in the right position was head-scratching.
Possible optimizations:
- Instead of copying and distributing the same root clock to the n counter cells, I could have just put the same clock block n times (once for each counter cell). This would actually be much simpler. But then I wouldn't be able to adjust it as easily by changing the clock at a single point... And I have an electronics background, and in a real circuit, that would be horribly wrong.
- Each segment has it own RS latch. This requires the lookup tables to output both R and S pulses. If we had a latch that would just toggle its state from a common input pulse, we could make the lookup tables half as big. There is such a latch for the PM dot, but it is huge, and I'm unable to come up with something more practical.
- Make the display smaller. But that wouldn't be as nice-looking. And it needed to be. Yes.
Does the clock have a fixed starting time? – FryAmTheEggman – 2016-08-04T19:10:33.517
Can The display be in binary? – None – 2016-08-04T19:15:21.290
1@FryAmTheEggman No, but the starting time shouldn't matter if the display state is cyclic. – Joe Z. – 2016-08-04T19:19:44.133
5@tuskiomi No, the display must be decimal. – Joe Z. – 2016-08-04T19:19:51.883
2I'm pretty sure this is B3/S23, but could you confirm or deny? – Conor O'Brien – 2016-08-04T19:29:07.273
Yes, B3/S23 is correct. – Joe Z. – 2016-08-04T19:32:38.847
1"from one change of minute to the next must take the same number of generations" assuming our digits change in a cascade-of-pixels fashion, can we simply ensure that the cascade starts and/or ends at the same time each minute? that is, 1 is narrower than 0, so 1 probably won't take as long to change. it can start, or middle, or end at the same time as 0, but not all 3 without a lot more work. – Sparr – 2016-08-04T20:02:26.873
2"They must also update in place — each new number must appear in the same place as the previous number." How do you define "in the same place", since the digits won't necessarily be rectangular. – Martin Ender – 2016-08-04T20:14:11.940
4how discernable must our decimal digits be? is "if you know what it is and you squint then you can tell the difference between 0 and 8" enough, or does it need to pass the "an anonymous bystander can tell what it is with no prompting" test? – Sparr – 2016-08-04T20:22:02.237
1@Sparr You can ensure that a cascade starts or ends, and an anonymous bystander should be able to tell without prompting. – Joe Z. – 2016-08-05T00:59:17.923
@MartinEnder There should be little to no movement of the bounding boxes of the digits. (You can make "1" right-aligned like a seven-segment display if you're implementing that, but otherwise the digits should all have roughly the same bounding box.) – Joe Z. – 2016-08-05T14:51:50.713
@MartinEnder I think he means it can't be a glider. – PyRulez – 2016-08-06T01:12:47.987
@PyRulez I built that rule to exclude the kinds of digits that appear in different positions and just get uncovered when the clock is on that digit. The digital clock display should look roughly like an actual digital clock display. – Joe Z. – 2016-08-06T15:52:16.157
1By Game of Life, do you mean the B3/S23 ruleset originally defined by Conway, or any Life-like cellular automata? Does it need to use a rectangular grid, or is a hexagonal grid acceptable? – Mego – 2016-08-07T23:14:33.447
2@Mego B3/S23, rectangular grid. – Joe Z. – 2016-08-08T07:57:18.190
2This is even harder than tetris as it seems. – Matthew Roh – 2017-01-23T23:46:50.157
3
this got posted on the Hackaday blog too : http://hackaday.com/2017/03/11/a-clock-created-with-conways-life/
– Anool Mahidharia – 2017-03-11T18:00:53.2572
How about this analogue clock⸮ https://copy.sh/life/?pattern=clock2&fps=1 (not made by me)
– copy – 2017-03-13T22:10:39.847So, its now answered, now you make a challenge harder than a clock but easier than tetris. what about... Pong? – Matthew Roh – 2017-03-17T11:53:31.043
@SIGSEGV A pong challenge already exists, so they could answer there.
– mbomb007 – 2017-04-24T17:26:44.9001The tetris challenge has been solved, you can update the top. – Rɪᴋᴇʀ – 2017-09-14T21:02:03.517