Java Jungle
(954 golfed)
Full of deep, twisting undergrowth, this is a forest not easily traversed.
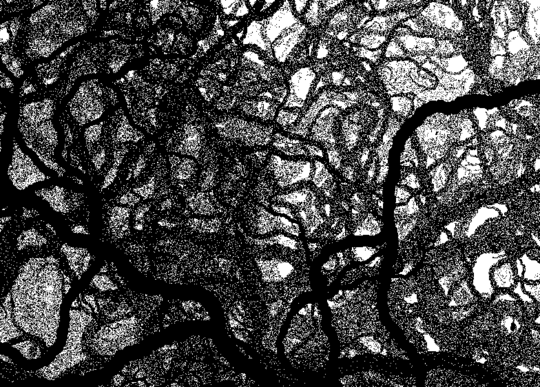
It's basically a fractal random walk with slowly shrinking, twisty vines. I draw 75 of them, gradually changing from white in the back to black up front. Then I dither the whole thing, shamelessly adapting Averroes' code here for that.
Golfed: (Just because others decided to)
import java.awt.*;import java.awt.image.*;import java.util.*;class P{static Random rand=new Random();public static void main(String[]a){float c=255;int i,j;Random rand=new Random();final BufferedImage m=new BufferedImage(800,600,BufferedImage.TYPE_INT_RGB);Graphics g=m.getGraphics();for(i=0;i++<75;g.setColor(new Color((int)c,(int)c,(int)c)),b(g,rand.nextInt(800),599,25+(rand.nextInt(21-10)),rand.nextInt(7)-3),c-=3.4);for(i=0;i<800;i++)for(j=0;j<600;j++)if(((m.getRGB(i,j)>>>16)&0xFF)/255d<rand.nextFloat()*.7+.05)m.setRGB(i,j,0);else m.setRGB(i,j,0xFFFFFF);new Frame(){public void paint(Graphics g){setSize(800,600);g.drawImage(m,0,0,null);}}.show();}static void b(Graphics g,float x,float y,float s,float a){if(s>1){g.fillOval((int)(x-s/2),(int)(y-s/2),(int)s,(int)s);s-=0.1;float n,t,u;for(int i=0,c=rand.nextInt(50)<1?2:1;i++<c;n=a+rand.nextFloat()-0.5f,n=n<-15?-15:n>15?15:n,t=x+s/2*(float)Math.cos(n),u=y-s/2*(float)Math.sin(n),b(g,t,u,s,n));}}}
Sane original code:
import java.awt.Color;
import java.awt.Graphics;
import java.awt.image.BufferedImage;
import java.util.Random;
import javax.swing.JFrame;
public class Paint {
static int minSize = 1;
static int startSize = 25;
static double shrink = 0.1;
static int branch = 50;
static int treeCount = 75;
static Random rand = new Random();
static BufferedImage img;
public static void main(String[] args) {
img = new BufferedImage(800,600,BufferedImage.TYPE_INT_ARGB);
forest(img);
dither(img);
new JFrame() {
public void paint(Graphics g) {
setSize(800,600);
g.drawImage(img,0,0,null);
}
}.show();
}
static void forest(BufferedImage img){
Graphics g = img.getGraphics();
for(int i=0;i<treeCount;i++){
int c = 255-(int)((double)i/treeCount*256);
g.setColor(new Color(c,c,c));
tree(g,rand.nextInt(800), 599, startSize+(rand.nextInt(21-10)), rand.nextInt(7)-3);
}
}
static void tree(Graphics g, double x, double y, double scale, double angle){
if(scale < minSize)
return;
g.fillOval((int)(x-scale/2), (int)(y-scale/2), (int)scale, (int)scale);
scale -= shrink;
int count = rand.nextInt(branch)==0?2:1;
for(int i=0;i<count;i++){
double newAngle = angle + rand.nextDouble()-0.5;
if(newAngle < -15) newAngle = -15;
if(newAngle > 15) newAngle = 15;
double nx = x + (scale/2)*Math.cos(newAngle);
double ny = y - (scale/2)*Math.sin(newAngle);
tree(g, nx, ny, scale, newAngle);
}
}
static void dither(BufferedImage img) {
for (int i=0;i<800;i++)
for (int j=0;j<600;j++) {
double lum = ((img.getRGB(i, j) >>> 16) & 0xFF) / 255d;
if (lum <= threshold[rand.nextInt(threshold.length)]-0.2)
img.setRGB(i, j, 0xFF000000);
else
img.setRGB(i, j, 0xFFFFFFFF);
}
}
static double[] threshold = { 0.25, 0.26, 0.27, 0.28, 0.29, 0.3, 0.31,
0.32, 0.33, 0.34, 0.35, 0.36, 0.37, 0.38, 0.39, 0.4, 0.41, 0.42,
0.43, 0.44, 0.45, 0.46, 0.47, 0.48, 0.49, 0.5, 0.51, 0.52, 0.53,
0.54, 0.55, 0.56, 0.57, 0.58, 0.59, 0.6, 0.61, 0.62, 0.63, 0.64,
0.65, 0.66, 0.67, 0.68, 0.69 };
}
One more? Okay! This one has the dithering tuned down a bit, so the blacks in front are much flatter.
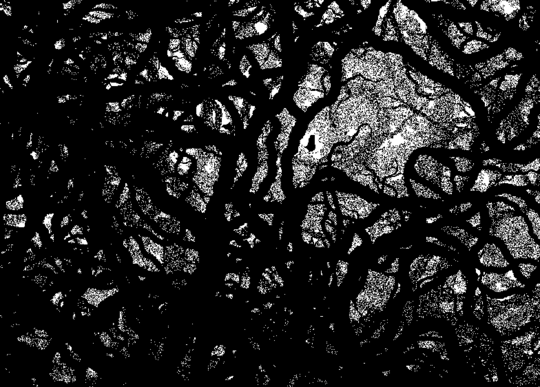
Unfortunately, the dither doesn't show the fine details of the vine layers. Here's a greyscale version, just for comparison:
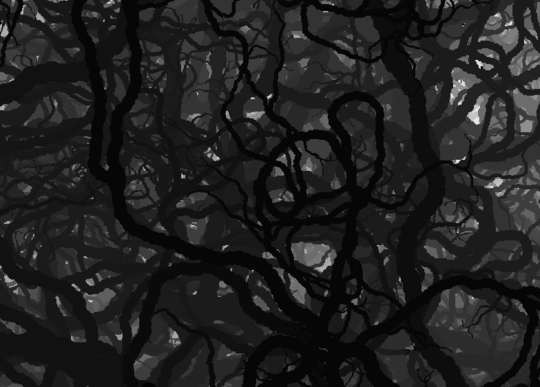
9This question appears to be off-topic because it is more an art contest rather than a programming contest. – ProgramFOX – 2014-08-06T16:50:18.103
19@ProgramFOX Isn't programming art? :) – Somnium – 2014-08-06T16:51:27.697
1
The question is about generating art, and the answers will likely compete by output, not by code. Example of a similar contest, which was also an art contest rather than a programming contest: http://codegolf.stackexchange.com/questions/20598/make-the-day-we-fight-back-logo
– ProgramFOX – 2014-08-06T16:54:49.7731@ProgramFOX I thought for this task various interesting algorithms can be used. – Somnium – 2014-08-06T17:03:39.077
Those algorithms will generate beautiful art, and better output will attract more votes, I think. Anyway, we'll see what other people think about it. – ProgramFOX – 2014-08-06T17:09:07.067
1
I think this question is more similar to this one: http://codegolf.stackexchange.com/questions/16826/lets-simulate-a-random-snowflake?rq=1 just for the randomness factor, and that one was well received.
– BrunoJ – 2014-08-06T17:13:32.4932@BrunoJ You're right, the community is getting tougher about closing art-based challenges. Some of my first answers (at the beginning of this year) were to questions similar to this one. I may mention it on Meta. – Level River St – 2014-08-06T17:35:58.753
15I, for one, would like to see some entries for this challenge, and am disappointed that it was put on hold. – Braden Best – 2014-08-06T21:08:03.560
@BrunoJ, the question you reference a) is less broad than this one (although it could still be improved considerably); b) was posted in the middle of a peak of activity which was characterised by a lot of poor questions and the absence due to holiday of some regulars. To get only 10 upvotes right then was not to be well received, and many questions which should have been closed managed not to be. – Peter Taylor – 2014-08-07T11:33:45.970
3I like this challenge. Answers that aren't in the spirit of it won't get upvoted as much, so what's the problem? – cjfaure – 2014-08-07T11:35:18.093
2
Special libraries for drawing trees/forests are disallowed
Why would those even exist? – cjfaure – 2014-08-07T12:55:16.7433@cjfaure For different purposes, for example for generating models and images for games. – Somnium – 2014-08-07T13:54:11.420
3"The question is about generating art, and the answers will likely compete by output, not by code" - this is a crazy argument. Its the code that generates the output. A question that asks for code that produces numbers is not considered to be too much of a maths challenge. – rdans – 2014-08-07T18:05:31.680
There are already answers to this question, and they clearly required programming - both effort and skill. Personally I would like to see questions with more restrictions to stimulate creative ways around them - this is broader than my personal preference. However, this question does have a number of restrictions and is not sufficiently broad to justify closing it. – trichoplax – 2014-08-08T02:59:45.163
The dithering is optional, is it not? – Lars Ebert – 2014-08-08T09:37:01.580
@LarsEbert Dithering is not mentioned in question at all (except example) so you may not use it. – Somnium – 2014-08-08T10:43:32.723
1http://algorithmicbotany.org/TreeSketch/ – Dr. belisarius – 2014-08-08T15:23:04.117
I assume that "you may not use it" means you are not required to use dithering. I can't see how there can be a ban on dithering as it can be implemented algorithmically from an algorithmically generated image, which meets the requirements of the question. – trichoplax – 2014-08-08T23:14:14.410
@githubphagocyte I meant only 2 colors - black and white. You are confusing with grayscale. – Somnium – 2014-08-11T13:56:01.823
@githubphagocyte If you didn't see I already have mentioned that in rules. – Somnium – 2014-08-11T14:30:15.003
Yes, I saw that from the start, but since it is common for people to read "black and white" as meaning the same thing as "greyscale" I'm just suggesting you could avoid confusion by changing "only 2 colors - black and white" to "only 2 colors - black and white (not greyscale)". Your wording is already perfectly clear, but since there are already people mistaking the meaning, it is worth adding the extra clarification to avoid arguments later and having to delete answers that clutter up your question. I like this question and don't want to see it fill up with off topic answers. – trichoplax – 2014-08-11T14:43:13.720
I also think making that clear might help avoid the close votes since some of the close voters also may not have realised that this isn't just a grayscale question. – trichoplax – 2014-08-11T14:49:53.260