Autoit 3 Script
$trol=ASCIIGenerate(4,1,1,0)&ASCIIGenerate(24,0,0,1)&ASCIIGenerate(2,1,1,0)&ASCIIGenerate(2,0,0,0)&ASCIIGenerate(24,1,0,0)&ASCIIGenerate(1,0,0,1)&ASCIIGenerate(2,1,1,0)&ASCIIGenerate(1,0,0,0)&ASCIIGenerate(26,1,0,0)&ASCIIGenerate(1,0,0,1)&ASCIIGenerate(1,1,1,0)&ASCIIGenerate(1,0,0,0)&ASCIIGenerate(28,1,0,0)&ASCIIGenerate(2,0,0,1)&ASCIIGenerate(1,1,1,0)&ASCIIGenerate(1,0,0,0)&ASCIIGenerate(30,1,0,0)&ASCIIGenerate(1,0,0,1)&ASCIIGenerate(1,1,1,0)&ASCIIGenerate(1,0,0,0)&ASCIIGenerate(30,1,0,0)&ASCIIGenerate(1,0,0,1)&ASCIIGenerate(2,1,1,0)&ASCIIGenerate(1,0,0,0)&ASCIIGenerate(28,1,0,0)&ASCIIGenerate(1,0,0,1)&ASCIIGenerate(2,1,1,0)&ASCIIGenerate(1,0,0,0)&ASCIIGenerate(26,1,0,0)&ASCIIGenerate(2,0,0,1)&ASCIIGenerate(2,1,1,0)&ASCIIGenerate(1,0,0,0)&ASCIIGenerate(21,1,0,0)&ASCIIGenerate(5,0,0,1)&ASCIIGenerate(2,1,1,0)&ASCIIGenerate(1,0,0,0)&ASCIIGenerate(12,1,0,0)&ASCIIGenerate(9,0,0,1)&ASCIIGenerate(3,1,1,0)&ASCIIGenerate(12,0,0,1)
RunWait("cmd /C @echo off&color F0&"&$trol&"pause > nul")
Func ASCIIGenerate($num,$char,$echo,$and)
Local $i=0
Local $str=""
If $echo=1 Then
$str&="echo "
EndIf
Do
Switch $char
Case 0
$str&="#"
Case 1
$str&=" "
EndSwitch
$i+=1
Until $i=$num
If $and=1 Then
$str&="&"
EndIf
Return $str
EndFunc
Output:
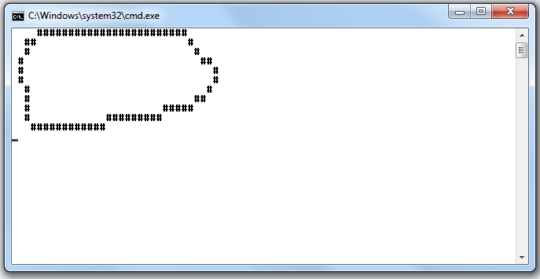
Holes exploited:
You can't have it stored in any image format or pre-rendered (including base64 data:// uri's and any form of ascii art).
The ASCII art is generated by the script on the fly. The script itself only has info on which and how many characters it must draw onscreen and when start a new line.
You can't store the image in any database.
The image must show in the screen. It can't output the code to a file or anything similar (/dev/null doesn't count and virtual screens/viewports also don't count).
The script doesn't create nor contain any files. When generated, the ASCII art is loaded onto the computer's RAM and then shown via CMD commands (without using any batch files, only cmd /c ).
The code must render the trolologram on the screen (minimum size of 90x90) and must be with a black border and white background. The rendered version can't "be invisible" or "hidden behind the screen" or similar.
The image displayed onscreen is way bigger than 90x90 and it's black on a white background.
1I believe this challenge is impossible, since all computer generated graphics have to be in some image format, but this is prohibited by the rules. – user12205 – 2014-02-20T21:41:17.443
1@ace OP should clarify but I believes he means that it should be drawn on the fly by the program, not pre-rendered and displayed. – Jason C – 2014-02-20T21:47:26.340
I hope it is more clear now. Sorry for the trouble. – Ismael Miguel – 2014-02-20T22:01:56.543
I think that this challenge is cool. Will think about something for it. – Victor Stafusa – 2014-02-20T22:27:15.857
Thank you. Any questions don't hesitate. And remember: this is code trolling. – Ismael Miguel – 2014-02-20T22:34:58.007
Are image formats that are also markup languages fair game (e.g. SVG)? – Jason C – 2014-02-20T22:58:33.903
No. It's still an image. But it's not hard to twist that to give a valid answer. – Ismael Miguel – 2014-02-20T23:14:24.173
NO! Don't touch the question! – Ismael Miguel – 2014-02-21T00:16:42.410
2The link at the top is in danger of becoming a circular reference, as this page rises up the Google ranks. Which might actually give us a loophole . . . – Neil Slater – 2014-02-21T13:42:10.230
sorry dude but that makes no sense to me... – Ismael Miguel – 2014-02-21T14:01:54.063
@NeilSlater It's already on the first page of results. – ntoskrnl – 2014-02-21T15:01:38.477
5If you create a challenge about trolling and expect serious answers, you're gonna have a bad time... – Nyx – 2014-02-21T18:49:06.593
Code-trolling is in the process of being removed, as per the official stance. This question has a very highly voted answer, recieved over 50% "keep" votes on the poll, and is fairly unique and different than the others, so I am locking it for historical significance.
– Doorknob – 2014-05-11T22:23:36.413