Wolfram Language 190 bytes
The following returns all of the graph edges in terms of the actual coordinates (assuming each mini-cube is 2 units at the edge and the Rubik's cube has its bottom left vertex at the origin).
t=Table;h[a_,b_,c_]:=t[{x,y,z},{a,1,5,2},{b,1,5,2},{c,0,6,6}];Partition[Sort[a=Cases[DeleteCases[Tuples[Flatten[{h[x,z,y],h[y,z,x],h[x,y,z]},3],{2}],{x_,x_}],x_/;ManhattanDistance@@x==2]],4]
(* output *)
{{{{0,1,1},{0,1,3}},{{0,1,1},{0,3,1}},{{0,1,1},{1,0,1}},{{0,1,1},{1,1,0}}},{{{0,1,3},{0,1,1}},{{0,1,3},{0,1,5}},{{0,1,3},{0,3,3}},{{0,1,3},{1,0,3}}},{{{0,1,5},{0,1,3}},{{0,1,5},{0,3,5}},{{0,1,5},{1,0,5}},{{0,1,5},{1,1,6}}},{{{0,3,1},{0,1,1}},{{0,3,1},{0,3,3}},{{0,3,1},{0,5,1}},{{0,3,1},{1,3,0}}},{{{0,3,3},{0,1,3}},{{0,3,3},{0,3,1}},{{0,3,3},{0,3,5}},{{0,3,3},{0,5,3}}},{{{0,3,5},{0,1,5}},{{0,3,5},{0,3,3}},{{0,3,5},{0,5,5}},{{0,3,5},{1,3,6}}},{{{0,5,1},{0,3,1}},{{0,5,1},{0,5,3}},{{0,5,1},{1,5,0}},{{0,5,1},{1,6,1}}},{{{0,5,3},{0,3,3}},{{0,5,3},{0,5,1}},{{0,5,3},{0,5,5}},{{0,5,3},{1,6,3}}},{{{0,5,5},{0,3,5}},{{0,5,5},{0,5,3}},{{0,5,5},{1,5,6}},{{0,5,5},{1,6,5}}},{{{1,0,1},{0,1,1}},{{1,0,1},{1,0,3}},{{1,0,1},{1,1,0}},{{1,0,1},{3,0,1}}},{{{1,0,3},{0,1,3}},{{1,0,3},{1,0,1}},{{1,0,3},{1,0,5}},{{1,0,3},{3,0,3}}},{{{1,0,5},{0,1,5}},{{1,0,5},{1,0,3}},{{1,0,5},{1,1,6}},{{1,0,5},{3,0,5}}},{{{1,1,0},{0,1,1}},{{1,1,0},{1,0,1}},{{1,1,0},{1,3,0}},{{1,1,0},{3,1,0}}},{{{1,1,6},{0,1,5}},{{1,1,6},{1,0,5}},{{1,1,6},{1,3,6}},{{1,1,6},{3,1,6}}},{{{1,3,0},{0,3,1}},{{1,3,0},{1,1,0}},{{1,3,0},{1,5,0}},{{1,3,0},{3,3,0}}},{{{1,3,6},{0,3,5}},{{1,3,6},{1,1,6}},{{1,3,6},{1,5,6}},{{1,3,6},{3,3,6}}},{{{1,5,0},{0,5,1}},{{1,5,0},{1,3,0}},{{1,5,0},{1,6,1}},{{1,5,0},{3,5,0}}},{{{1,5,6},{0,5,5}},{{1,5,6},{1,3,6}},{{1,5,6},{1,6,5}},{{1,5,6},{3,5,6}}},{{{1,6,1},{0,5,1}},{{1,6,1},{1,5,0}},{{1,6,1},{1,6,3}},{{1,6,1},{3,6,1}}},{{{1,6,3},{0,5,3}},{{1,6,3},{1,6,1}},{{1,6,3},{1,6,5}},{{1,6,3},{3,6,3}}},{{{1,6,5},{0,5,5}},{{1,6,5},{1,5,6}},{{1,6,5},{1,6,3}},{{1,6,5},{3,6,5}}},{{{3,0,1},{1,0,1}},{{3,0,1},{3,0,3}},{{3,0,1},{3,1,0}},{{3,0,1},{5,0,1}}},{{{3,0,3},{1,0,3}},{{3,0,3},{3,0,1}},{{3,0,3},{3,0,5}},{{3,0,3},{5,0,3}}},{{{3,0,5},{1,0,5}},{{3,0,5},{3,0,3}},{{3,0,5},{3,1,6}},{{3,0,5},{5,0,5}}},{{{3,1,0},{1,1,0}},{{3,1,0},{3,0,1}},{{3,1,0},{3,3,0}},{{3,1,0},{5,1,0}}},{{{3,1,6},{1,1,6}},{{3,1,6},{3,0,5}},{{3,1,6},{3,3,6}},{{3,1,6},{5,1,6}}},{{{3,3,0},{1,3,0}},{{3,3,0},{3,1,0}},{{3,3,0},{3,5,0}},{{3,3,0},{5,3,0}}},{{{3,3,6},{1,3,6}},{{3,3,6},{3,1,6}},{{3,3,6},{3,5,6}},{{3,3,6},{5,3,6}}},{{{3,5,0},{1,5,0}},{{3,5,0},{3,3,0}},{{3,5,0},{3,6,1}},{{3,5,0},{5,5,0}}},{{{3,5,6},{1,5,6}},{{3,5,6},{3,3,6}},{{3,5,6},{3,6,5}},{{3,5,6},{5,5,6}}},{{{3,6,1},{1,6,1}},{{3,6,1},{3,5,0}},{{3,6,1},{3,6,3}},{{3,6,1},{5,6,1}}},{{{3,6,3},{1,6,3}},{{3,6,3},{3,6,1}},{{3,6,3},{3,6,5}},{{3,6,3},{5,6,3}}},{{{3,6,5},{1,6,5}},{{3,6,5},{3,5,6}},{{3,6,5},{3,6,3}},{{3,6,5},{5,6,5}}},{{{5,0,1},{3,0,1}},{{5,0,1},{5,0,3}},{{5,0,1},{5,1,0}},{{5,0,1},{6,1,1}}},{{{5,0,3},{3,0,3}},{{5,0,3},{5,0,1}},{{5,0,3},{5,0,5}},{{5,0,3},{6,1,3}}},{{{5,0,5},{3,0,5}},{{5,0,5},{5,0,3}},{{5,0,5},{5,1,6}},{{5,0,5},{6,1,5}}},{{{5,1,0},{3,1,0}},{{5,1,0},{5,0,1}},{{5,1,0},{5,3,0}},{{5,1,0},{6,1,1}}},{{{5,1,6},{3,1,6}},{{5,1,6},{5,0,5}},{{5,1,6},{5,3,6}},{{5,1,6},{6,1,5}}},{{{5,3,0},{3,3,0}},{{5,3,0},{5,1,0}},{{5,3,0},{5,5,0}},{{5,3,0},{6,3,1}}},{{{5,3,6},{3,3,6}},{{5,3,6},{5,1,6}},{{5,3,6},{5,5,6}},{{5,3,6},{6,3,5}}},{{{5,5,0},{3,5,0}},{{5,5,0},{5,3,0}},{{5,5,0},{5,6,1}},{{5,5,0},{6,5,1}}},{{{5,5,6},{3,5,6}},{{5,5,6},{5,3,6}},{{5,5,6},{5,6,5}},{{5,5,6},{6,5,5}}},{{{5,6,1},{3,6,1}},{{5,6,1},{5,5,0}},{{5,6,1},{5,6,3}},{{5,6,1},{6,5,1}}},{{{5,6,3},{3,6,3}},{{5,6,3},{5,6,1}},{{5,6,3},{5,6,5}},{{5,6,3},{6,5,3}}},{{{5,6,5},{3,6,5}},{{5,6,5},{5,5,6}},{{5,6,5},{5,6,3}},{{5,6,5},{6,5,5}}},{{{6,1,1},{5,0,1}},{{6,1,1},{5,1,0}},{{6,1,1},{6,1,3}},{{6,1,1},{6,3,1}}},{{{6,1,3},{5,0,3}},{{6,1,3},{6,1,1}},{{6,1,3},{6,1,5}},{{6,1,3},{6,3,3}}},{{{6,1,5},{5,0,5}},{{6,1,5},{5,1,6}},{{6,1,5},{6,1,3}},{{6,1,5},{6,3,5}}},{{{6,3,1},{5,3,0}},{{6,3,1},{6,1,1}},{{6,3,1},{6,3,3}},{{6,3,1},{6,5,1}}},{{{6,3,3},{6,1,3}},{{6,3,3},{6,3,1}},{{6,3,3},{6,3,5}},{{6,3,3},{6,5,3}}},{{{6,3,5},{5,3,6}},{{6,3,5},{6,1,5}},{{6,3,5},{6,3,3}},{{6,3,5},{6,5,5}}},{{{6,5,1},{5,5,0}},{{6,5,1},{5,6,1}},{{6,5,1},{6,3,1}},{{6,5,1},{6,5,3}}},{{{6,5,3},{5,6,3}},{{6,5,3},{6,3,3}},{{6,5,3},{6,5,1}},{{6,5,3},{6,5,5}}},{{{6,5,5},{5,5,6}},{{6,5,5},{5,6,5}},{{6,5,5},{6,3,5}},{{6,5,5},{6,5,3}}}}
The work of generating the points on each external facet is done by the function, h
.
It has to be called 3 times to generate the points at x=0, x=6; y=0, y=6; and z=0,z=6.
Each facet point that is a Manhattan distance of 2 units from another will be connected to the respective point.
We can display the graph edges visually by the following; a
is the list of graph edges that are represented below as arrows.
Graphics3D[{Arrowheads[.02],Arrow/@a},Boxed->False,Axes-> True]
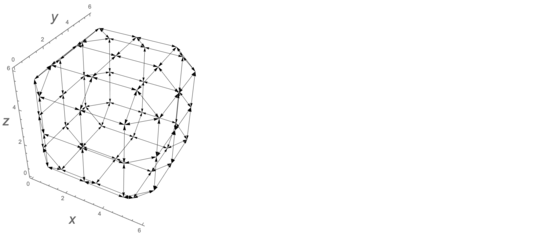
The following shows the Rubik's cube, the points on the external facets, and 8 graph edges.
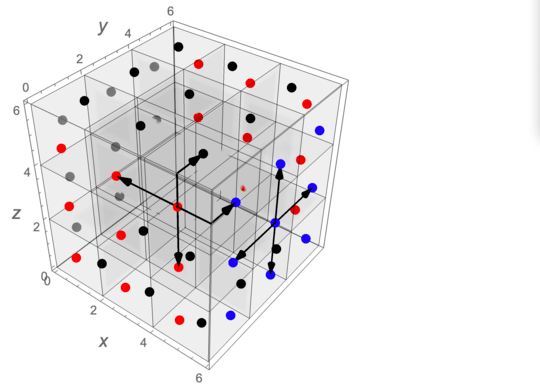
Red dots are located on facets at y = 0 and y = 6; blue and gray dots are on facets at x = 6 and x = 0, respectively; black dots are on facets at z=6 and z=0.
@jimmy23013 using π is very nice :) thank you! – ngn – 2019-05-19T23:48:23.323
54x54 matrix... thats impressive – don bright – 2019-05-27T20:29:11.950