?'+)=:!@/*"*'6/{=+'+}/{
Try it online!
Explanation
Unfolded:
? ' + )
= : ! @ /
* " * ' 6 /
{ = + ' + } /
{ . . . . .
. . . . .
. . . .
This is really just a linear program with the /
used for some redirection. The linear code is:
?'+){=+'+}*"*'6{=:!@
Which computes n (n+1) (2n+1) / 6. It uses the following memory edges:
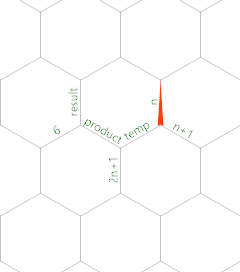
Where the memory point (MP) starts on the edge labelled n, pointing north.
? Read input into edge labelled 'n'.
' Move MP backwards onto edge labelled 'n+1'.
+ Copy 'n' into 'n+1'.
) Increment the value (so that it actually stores the value n+1).
{= Move MP forwards onto edge labelled 'temp' and turn around to face
edges 'n' and 'n+1'.
+ Add 'n' and 'n+1' into edge 'temp', so that it stores the value 2n+1.
' Move MP backwards onto edge labelled '2n+1'.
+ Copy the value 2n+1 into this edge.
} Move MP forwards onto 'temp' again.
* Multiply 'n' and 'n+1' into edge 'temp', so that it stores the value
n(n+1).
" Move MP backwards onto edge labelled 'product'.
* Multiply 'temp' and '2n+1' into edge 'product', so that it stores the
value n(n+1)(2n+1).
' Move MP backwards onto edge labelled '6'.
6 Store an actual 6 there.
{= Move MP forwards onto edge labelled 'result' and turn around, so that
the MP faces edges 'product' and '6'.
: Divide 'product' by '6' into 'result', so that it stores the value
n(n+1)(2n+1)/6, i.e. the actual result.
! Print the result.
@ Terminate the program.
In theory it might be possible to fit this program into side-length 3, because the /
aren't needed for the computation, the :
can be reused to terminate the program, and some of the '"=+*{
might be reusable as well, bringing the number of required commands below 19 (the maximum for side-length 3). I doubt it's possible to find such a solution by hand though, if one exists at all.
2Fun observation from OEIS: This sequence contains exactly two perfect squares:
f(1) == 1 * 1 (1)
, andf(24) == 70 * 70 (4900)
. – James – 2017-10-17T16:31:10.910May we begin the sequence at
f(1) = 1
? – Emigna – 2017-10-18T06:10:34.810@Emigna sorry but no, you need to start from
f(0) = 0
. i've pointed out that to the few answers that failed that requirement – Felipe Nardi Batista – 2017-10-18T09:44:20.383The
f(0) = 0
requirement ruined a few of my solutions :( – ATaco – 2017-10-19T05:19:11.237