Mathematica 116 114 bytes
With several bytes saved thanks to Misha Lavrov.
Last@FindPath[Graph[Rule@@@Cases[Tuples[Tuples[{0,1},{l=Length@#}],{2}],x_/;Count[Plus@@x,1]==1]],##,{1,2^l},Alll]&
Input (8 dimensions)
[{1,0,0,1,0,0,0,1},{1,1,0,0,0,0,1,1}]//AbsoluteTiming
Output (length = 254, after 1.82 seconds)
{1.82393, {{1, 0, 0, 1, 0, 0, 0, 1}, {0, 0, 0, 1, 0, 0, 0, 1}, {0, 0, 0, 0, 0, 0, 0, 1}, {0, 0, 0, 0, 0, 0, 0, 0}, {0, 0, 0, 0, 0, 0, 1, 0}, {0, 0,0, 0, 0, 0, 1, 1}, {0, 0, 0, 0, 0, 1, 1, 1}, {0, 0, 0, 0, 0, 1, 0, 1}, {0, 0, 0, 0, 0, 1, 0, 0}, {0, 0, 0, 0, 0, 1, 1, 0}, {0, 0, 0, 0,1, 1, 1,0}, {0, 0, 0, 0, 1, 0, 1, 0}, {0, 0, 0, 0, 1, 0, 0, 0}, {0, 0, 0, 0, 1, 0, 0, 1}, {0, 0, 0, 0, 1, 0, 1, 1}, {0, 0, 0, 0,1, 1, 1, 1}, {0, 0, 0, 0, 1, 1, 0, 1}, {0, 0, 0, 0, 1, 1, 0, 0}, {0, 0, 0, 1, 1, 1, 0, 0}, {0, 0, 0, 1, 0, 1, 0, 0}, {0, 0, 0, 1,0, 0, 0, 0}, {0, 0, 0, 1, 0, 0, 1, 0}, {0, 0, 0, 1, 0, 0, 1, 1}, {0, 0, 0, 1, 0, 1, 1, 1}, {0, 0, 0, 1, 0, 1, 0, 1}, {0, 0, 0, 1, 1, 1, 0, 1}, {0, 0, 0, 1, 1, 0, 0, 1}, {0, 0, 0, 1, 1, 0, 0, 0}, {0, 0, 0, 1, 1, 0, 1, 0}, {0, 0, 0, 1, 1, 0, 1, 1}, {0, 0, 0, 1,1, 1, 1, 1}, {0, 0, 0, 1, 1, 1, 1, 0}, {0, 0, 0, 1, 0, 1, 1, 0}, {0, 0, 1, 1, 0, 1, 1, 0}, {0, 0, 1, 0, 0, 1, 1, 0}, {0, 0, 1, 0,0, 0, 1, 0}, {0, 0, 1, 0, 0, 0, 0, 0}, {0, 0, 1, 0, 0, 0, 0, 1}, {0, 0, 1, 0, 0, 0, 1, 1}, {0, 0, 1, 0, 0, 1, 1, 1}, {0, 0, 1, 0,0, 1, 0, 1}, {0, 0, 1, 0, 0, 1, 0, 0}, {0, 0, 1, 0, 1, 1, 0, 0}, {0, 0, 1, 0, 1, 0, 0, 0}, {0, 0, 1, 0, 1, 0, 0, 1}, {0, 0, 1, 0,1, 0, 1, 1}, {0, 0, 1, 0, 1, 0, 1, 0}, {0, 0, 1, 0, 1, 1, 1, 0}, {0, 0, 1, 0, 1, 1, 1, 1}, {0, 0, 1, 0, 1, 1, 0, 1}, {0, 0, 1, 1,1, 1, 0, 1}, {0, 0, 1, 1, 0, 1, 0, 1}, {0, 0, 1, 1, 0, 0, 0, 1}, {0, 0, 1, 1, 0, 0, 0, 0}, {0, 0, 1, 1, 0, 0, 1, 0}, {0, 0, 1, 1,0, 0, 1, 1}, {0, 0, 1, 1, 0, 1, 1,1}, {0, 0, 1, 1, 1, 1, 1, 1}, {0, 0, 1, 1, 1, 0, 1, 1}, {0, 0, 1, 1, 1, 0, 0, 1}, {0, 0, 1, 1,1, 0, 0, 0}, {0, 0, 1, 1, 1, 0, 1, 0}, {0, 0, 1, 1, 1, 1, 1, 0}, {0, 0, 1, 1, 1, 1, 0, 0}, {0, 0, 1, 1, 0, 1, 0, 0}, {0, 1, 1, 1,0, 1, 0, 0}, {0, 1, 0, 1, 0, 1, 0, 0}, {0, 1, 0, 0, 0, 1, 0, 0}, {0, 1, 0, 0, 0, 0, 0, 0}, {0, 1, 0, 0, 0, 0, 0, 1}, {0, 1, 0, 0,0, 0, 1, 1}, {0, 1, 0, 0, 0, 0, 1, 0}, {0, 1, 0, 0, 0, 1, 1, 0}, {0, 1, 0, 0, 0, 1, 1, 1}, {0, 1, 0, 0, 0, 1, 0, 1}, {0, 1, 0, 0,1, 1, 0, 1}, {0, 1, 0, 0, 1, 0, 0, 1}, {0, 1, 0, 0, 1, 0, 0, 0}, {0, 1, 0, 0, 1, 0, 1, 0}, {0, 1, 0, 0, 1, 0, 1, 1}, {0, 1, 0, 0,1, 1, 1, 1}, {0, 1, 0, 0, 1, 1, 1, 0}, {0, 1, 0, 0, 1, 1, 0,0}, {0, 1, 0, 1, 1, 1, 0, 0}, {0, 1, 0, 1, 1, 0, 0, 0}, {0, 1, 0, 1,0, 0, 0, 0}, {0, 1, 0, 1, 0, 0, 0, 1}, {0, 1, 0, 1, 0, 0, 1, 1}, {0, 1, 0, 1, 0, 0, 1, 0}, {0, 1, 0, 1, 0, 1, 1, 0}, {0, 1, 0, 1,0, 1, 1, 1}, {0, 1, 0, 1, 0, 1, 0, 1}, {0, 1, 0, 1, 1, 1, 0, 1}, {0, 1, 0, 1, 1, 0, 0, 1}, {0, 1, 0, 1, 1, 0, 1, 1}, {0, 1, 0, 1,1, 0, 1, 0}, {0, 1, 0, 1, 1, 1, 1, 0}, {0, 1, 0, 1, 1, 1, 1, 1}, {0, 1, 1, 1, 1, 1, 1, 1}, {0, 1, 1, 0, 1, 1, 1, 1}, {0, 1, 1, 0,0, 1, 1, 1}, {0, 1, 1, 0, 0, 0, 1, 1}, {0, 1, 1, 0, 0, 0, 0, 1}, {0, 1, 1, 0, 0, 0, 0, 0}, {0, 1, 1, 0, 0, 0, 1, 0}, {0, 1, 1, 0,0, 1, 1, 0}, {0, 1, 1, 0, 0, 1, 0, 0}, {0, 1, 1, 0, 0, 1, 0, 1}, {0, 1, 1, 0, 1, 1, 0, 1}, {0, 1, 1, 0, 1, 0, 0, 1}, {0, 1, 1, 0,1, 0, 0, 0}, {0, 1, 1, 0, 1, 0, 1, 0}, {0, 1, 1, 0, 1, 0, 1, 1}, {0, 1, 1, 1, 1, 0, 1, 1}, {0, 1, 1, 1, 0, 0, 1, 1}, {0, 1, 1, 1,0, 0, 0, 1}, {0, 1, 1, 1, 0, 0, 0, 0}, {0, 1, 1, 1, 0, 0, 1, 0}, {0, 1, 1, 1, 0, 1, 1, 0}, {0, 1, 1, 1, 0, 1, 1, 1}, {0, 1, 1, 1,0, 1, 0, 1}, {0, 1, 1, 1, 1, 1, 0, 1}, {0, 1, 1, 1, 1, 0, 0, 1}, {0, 1, 1, 1, 1, 0, 0, 0}, {0, 1, 1, 1, 1, 0, 1, 0}, {0, 1, 1, 1,1, 1, 1, 0}, {0, 1, 1, 0, 1, 1, 1, 0}, {0, 1, 1, 0, 1, 1, 0, 0}, {0, 1, 1, 1, 1, 1, 0, 0}, {1, 1, 1, 1, 1, 1, 0, 0}, {1, 0, 1, 1,1, 1, 0, 0}, {1, 0, 0, 1, 1, 1, 0, 0}, {1, 0, 0, 0, 1, 1, 0, 0}, {1, 0, 0, 0, 0, 1, 0, 0}, {1, 0, 0, 0, 0, 0, 0, 0}, {1, 0, 0, 0,0, 0, 0, 1}, {1, 0, 0, 0, 0, 0, 1, 1}, {1, 0, 0, 0, 0, 0, 1, 0}, {1, 0, 0, 0, 0, 1, 1, 0}, {1, 0, 0, 0, 0, 1, 1, 1}, {1, 0, 0, 0,0, 1, 0, 1}, {1, 0, 0, 0, 1, 1, 0, 1}, {1, 0, 0, 0, 1, 0, 0, 1}, {1, 0, 0, 0, 1, 0, 0, 0}, {1, 0, 0, 0, 1, 0, 1, 0}, {1, 0, 0, 0,1, 0, 1, 1}, {1, 0, 0, 0, 1, 1, 1, 1}, {1, 0, 0, 0, 1, 1, 1, 0}, {1, 0, 0, 1, 1, 1, 1, 0}, {1, 0, 0, 1, 0, 1, 1, 0}, {1, 0, 0, 1,0, 0, 1, 0}, {1, 0, 0, 1, 0, 0, 0, 0}, {1, 0, 0, 1, 0, 1, 0, 0}, {1, 0, 0, 1, 0, 1, 0, 1}, {1, 0, 0, 1, 0, 1, 1, 1}, {1, 0, 0, 1,0, 0, 1, 1}, {1, 0, 0, 1, 1, 0, 1, 1}, {1, 0, 0, 1, 1, 0, 0, 1}, {1, 0, 0, 1, 1, 0, 0, 0}, {1, 0, 0, 1, 1, 0, 1, 0}, {1, 0, 1, 1,1, 0, 1, 0}, {1, 0, 1, 0, 1, 0, 1, 0}, {1, 0, 1, 0, 0, 0, 1, 0}, {1, 0, 1, 0, 0, 0, 0, 0}, {1, 0, 1, 0, 0, 0, 0, 1}, {1, 0, 1, 0,0, 0, 1, 1}, {1, 0, 1, 0, 0, 1, 1, 1}, {1, 0, 1, 0, 0, 1, 0, 1}, {1, 0, 1, 0, 0, 1, 0, 0}, {1, 0, 1, 0, 0, 1, 1, 0}, {1, 0, 1, 0,1, 1, 1, 0}, {1, 0, 1, 0, 1, 1, 0, 0}, {1, 0, 1, 0, 1, 0, 0, 0}, {1, 0, 1, 0, 1, 0, 0, 1}, {1, 0, 1, 0, 1, 0, 1, 1}, {1, 0, 1, 0,1, 1, 1, 1}, {1, 0, 1, 0, 1, 1, 0, 1}, {1, 0, 1, 1, 1, 1, 0, 1}, {1, 0, 0, 1, 1, 1, 0, 1}, {1, 0, 0, 1, 1, 1, 1, 1}, {1, 0, 1, 1,1, 1, 1, 1}, {1, 0, 1, 1, 0, 1, 1, 1}, {1, 0, 1, 1, 0, 0, 1, 1}, {1, 0, 1, 1, 0, 0, 0, 1}, {1, 0, 1, 1, 0, 0, 0, 0}, {1, 0, 1, 1,0, 0, 1, 0}, {1, 0, 1, 1, 0, 1, 1, 0}, {1, 0, 1, 1, 0, 1, 0, 0}, {1, 0, 1, 1, 0, 1, 0, 1}, {1, 1, 1, 1, 0, 1, 0, 1}, {1, 1, 0, 1,0, 1, 0, 1}, {1, 1, 0, 0, 0, 1, 0,1}, {1, 1, 0, 0, 0, 0, 0, 1}, {1, 1, 0, 0, 0, 0, 0, 0}, {1, 1, 0, 0, 0, 0, 1, 0}, {1, 1, 0, 0,0, 1, 1, 0}, {1, 1, 0, 0, 0, 1, 0, 0}, {1, 1, 0, 0, 1, 1, 0, 0}, {1, 1, 0, 0, 1, 0, 0, 0}, {1, 1, 0, 0, 1, 0, 0, 1}, {1, 1, 0, 0,1, 0, 1, 1}, {1, 1, 0, 0, 1, 0, 1, 0}, {1, 1, 0, 0, 1, 1, 1, 0}, {1, 1, 0, 0, 1, 1, 1, 1}, {1, 1, 0, 0, 0, 1, 1, 1}, {1, 1, 0, 1,0, 1, 1, 1}, {1, 1, 0, 1, 0, 0, 1, 1}, {1, 1, 0, 1, 0, 0, 0, 1}, {1, 1, 0, 1, 0, 0, 0, 0}, {1, 1, 0, 1, 0, 0, 1, 0}, {1, 1, 0, 1,0, 1, 1, 0}, {1, 1, 0, 1, 0, 1, 0, 0}, {1, 1, 0, 1, 1, 1, 0, 0}, {1, 1, 0, 1, 1, 0, 0, 0}, {1, 1, 0, 1, 1, 0, 0, 1}, {1, 1, 0, 1,1, 0, 1, 1}, {1, 1, 0, 1, 1, 0, 1, 0}, {1, 1, 0, 1, 1, 1, 1, 0}, {1, 1, 0, 1, 1, 1, 1, 1}, {1, 1, 0, 1, 1, 1, 0, 1}, {1, 1, 0, 0,1, 1, 0, 1}, {1, 1, 1, 0, 1, 1, 0, 1}, {1, 1, 1, 0, 0, 1, 0, 1}, {1, 1, 1, 0, 0, 0, 0, 1}, {1, 1, 1, 0, 0, 0, 0, 0}, {1, 1, 1, 0,0, 0, 1, 0}, {1, 1, 1, 0, 0, 1, 1, 0}, {1, 1, 1, 0, 0, 1, 0, 0}, {1, 1, 1, 0, 1, 1, 0, 0}, {1, 1, 1, 0, 1, 0, 0, 0}, {1, 1, 1, 0,1, 0, 0, 1}, {1, 1, 1, 0, 1, 0, 1, 1}, {1, 1, 1, 0, 1, 0, 1, 0}, {1, 1, 1, 0, 1, 1, 1, 0}, {1, 1, 1, 0, 1, 1, 1, 1}, {1, 1, 1, 0,0, 1, 1, 1}, {1, 1, 1, 1, 0, 1, 1, 1}, {1, 1, 1, 1, 0, 1, 1, 0}, {1, 1, 1, 1, 0, 0, 1, 0}, {1, 1, 1, 1, 0, 0, 0, 0}, {1, 1, 1, 1,0, 0, 0, 1}, {1, 1, 1, 1, 1, 0, 0, 1}, {1, 1, 1, 1, 1, 1, 0, 1}, {1, 1, 1, 1, 1, 1, 1, 1}, {1, 1, 1, 1, 1, 1, 1, 0}, {1, 1, 1, 1,1, 0, 1, 0}, {1, 1, 1, 1, 1, 0, 0, 0}, {1, 0, 1, 1, 1, 0, 0, 0}, {1, 0, 1, 1, 1, 0, 0, 1}, {1, 0, 1, 1, 1, 0, 1, 1}, {1, 1, 1, 1,1, 0, 1, 1}, {1, 1, 1, 1, 0, 0, 1, 1}, {1, 1, 1, 0, 0, 0, 1, 1}, {1, 1, 0, 0, 0, 0, 1, 1}}}
Tuples[{0,1},{l=Length@#}],{2}]
& generates the numbers 0...8 as binary lists.
The outer Tuples...{2}
produces all ordered pairs of those binary numbers.
Plus@@x
sums each of the pairs, generating triplets of 0, 1.
Cases....Count[Plus@@x, 1]==1
returns all of the sums that contain a single 1. These arise when the two original binary numbers are connected by an edge.
Rules
connects the vertices of the graph, each vertex being a binary number.
Graph
creates a graph corresponding to said vertices and edges.
FindPath
finds up to 2^n paths connecting vertex a to vertex b, the given numbers.
Last
takes the longest of these paths.
For three dimensions, the graph can be represented in a plane as shown here:
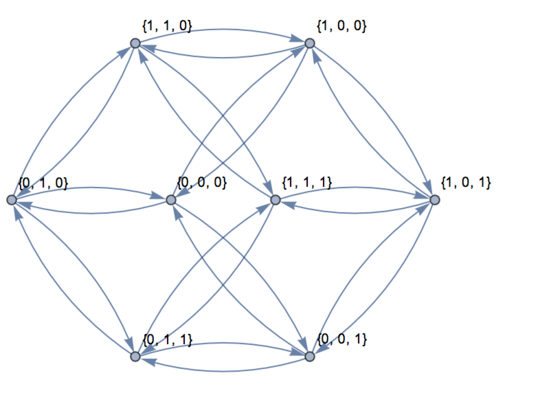
For the input, {0,0,0}, {1,1,1}
, the following is output:
{{{0, 0, 0}, {0, 0, 1}, {0, 1, 1}, {0, 1, 0}, {1, 1, 0}, {1, 0,
0}, {1, 0, 1}, {1, 1, 1}}}
This path can be found in the above graph.
It can also be conceived as the following path in 3-space,
where each vertex corresponds to a point {x,y,z}
. {0,0,0} represents the origin and {1,1,1} represents the "opposite" point in a unit cube.
So the solution path corresponds to a traversal of edges along the unit cube. In this case, the path is Hamiltonian: it visits each vertex one time (i.e. with no crossings and no vertices omitted).
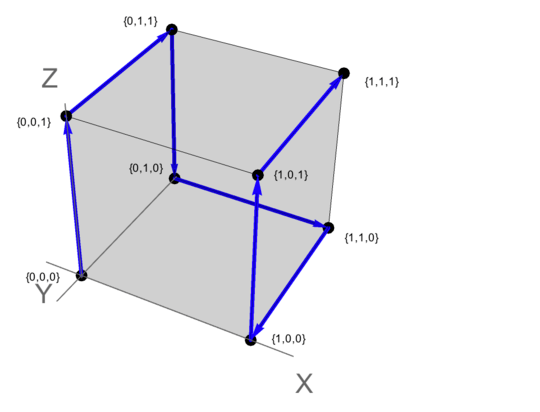
1Can we also take boolean values instead of ones and zeros? – flawr – 2017-09-30T19:59:47.707
@flawr Sure, that's fine. – Misha Lavrov – 2017-09-30T20:05:02.997
May we assume we will not receive two equal bit-strings (or that we may do anything if so)? – Jonathan Allan – 2017-09-30T23:00:23.467
1@JonathanAllan Yes, let's assume that the bit-strings are not equal. – Misha Lavrov – 2017-09-30T23:04:44.610
Related – Leaky Nun – 2017-10-01T00:14:00.877
Never mind that comment, close vote retracted. – caird coinheringaahing – 2017-10-01T00:21:59.757
@LeakyNun The Gray code gives us the "go from
00...00
to00...01
" case of this problem. (And, of course, it can be tweaked for other cases where the start and end are adjacent.) But it would be interesting if there's a more general solution along those lines. – Misha Lavrov – 2017-10-01T00:24:08.080Can I take input as
0b
input... – Stan Strum – 2017-10-01T17:59:31.170@StanStrum Assuming that you mean "as binary integers" then I've said in the rules that you can't. – Misha Lavrov – 2017-10-01T18:01:21.920