Grid size 6 (91 bytes)
? { 2 . . <
/ = { * = \ "
. & . . . { _ '
. . { . . } ' * 2
_ } { / . } & . ! "
. > % . . < | . . @ |
\ . . \ . | ~ . . 3
. . " . } . " . "
. & . \ = & / 1
\ = { : = } .
[ = { { { <
Compact version
?{2..</={*=\".&...{_'..{..}'*2_}{/.}&.!".>%..<|..@|\..\.|~..3..".}.".".&.\=&/1\={:=}.[={{{<
Grid size 7 (112 bytes)
? { 2 " ' 2 <
/ { * \ / : \ "
. = . = . = | . 3
/ & / { . . } { . "
. > $ } { { } / = . 1
_ . . } . . _ . . & . {
. > % < . . ~ & . . " . |
| . . } - " . ' . @ | {
. . . = & . . * ! . {
. . . _ . . . _ . =
> 1 . . . . . < [
. . . . . . . .
. . . . . . .
Try it online!
Compact Version:
?{2"'2</{*\/:\".=.=.=|.3/&/{..}{.".>$}{{}/=.1_..}.._..&.{.>%<..~&..".||..}-".'.@|{...=&..*!.{..._..._.=>1.....<[
Ungolfed Version for better readability:
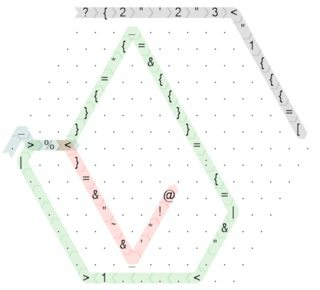
Approximate Memory Layout
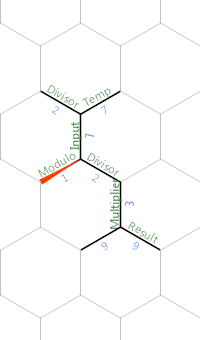
Grey Path (Memory initialization)
? Read input as integer (Input)
{ Move to memory edge "Divisor left"
2 Set current memory edge to 2
" ' Move to memory edge "Divisor right"
2 Set current memory edge to 2
" Move to memory edge "Multiplier"
3 Set current memory edge to 3
" Move to memory edge "Temp 2"
1 Set current memory edge to 1
{ { { Move to memory edge "Modulo"
= Turn memory pointer around
[ Continue with next instruction pointer
Loop entry
% Set "Modulo" to Input mod Divisor
< Branch depending on result
Green Path (value is still divisible by 2)
} } { Move to memory edge "Result"
= Turn memory pointer around
* Set "Result" to "Temp 2" times "Multiplier" (3)
{ = & Copy "Result" into "Temp2"
{ { } } = Move to "Temp"
: Set "Temp" to Input / Divisor (2)
{ = & Copy "Temp" back to "Input"
" Move back to "Modulo"
Red Path (value is no longer divisible by 2)
} = & " ~ & ' Drag what's left of "Input" along to "Multiplier"
* Multiply "Multiplier" with "Temp 2"
! @ Output result, exit program
10Talk about right tool for the job.. – Kevin Cruijssen – 2017-05-05T07:02:58.527
23"Don't upvote trivial solutions that only uses a simple builtin." Well, in this case: Knowing that there's a language "Fractran" that has a single builtin that solves this specific task is by itself impressive. – Stewie Griffin – 2017-05-05T08:51:16.570
Does this do primer factorization? – Pandya – 2017-05-05T12:09:17.553
@Pandya: Some Fractran implementations use prime factorization internally (as an optimization), but they don't have to; the language operates on factors, not prime factors. (It just happens that 2 and 3 are prime.) – None – 2017-05-05T12:23:25.667
3
Related SO code golf (pre-PPCG): Write a Fractran interpreter.
– hobbs – 2017-05-08T05:29:33.573Why does this exist? Its confusing. What was Conway looking for/studying? – Brain Guider – 2017-05-08T10:31:59.353
1@AnderBiguri: Likely looking for a Turing-complete language that's very simple/easy to implement. Fractran is really elegant as Turing tarpits go; most have a lot more rough edges, special cases, or details that could be changed without making a large difference. – None – 2017-05-08T13:25:54.983
3@AnderBiguri It looks like it came out of his studies of the Collatz conjecture; he proved that a generalization of Collatz is equivalent to Fractran and that Fractran is Turing-complete, therefore generalized Collatz is undecidable. – hobbs – 2017-05-08T18:00:38.247