7
2
XKCD Comic: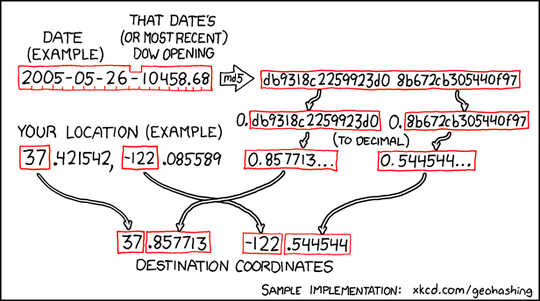
Goal:
Given a date, the current Dow Opening, and your current coordinates as a rounded integer, produce a "geohash."
Input:
Input through any reasonable means (STDIN, function argument, flag, etc.) the following:
- The current date. This does necessarily have to be the date of the system's clock, so assume that the input is correct.
- The most recent Dow Opening (must support at least 2 decimal places)
- The floor of your current latitude and longitude.
To make input easier, you can input through any reasonable structured format. Here are some examples (you may create your own):
["2005","05","26","10458.68","37",-122"]
2005-05-26,10458.68,37,-122
05-26-05 10458.68 37 -122
Algorithm:
Given your arguments, you must perform the "geohash" algorithm illustrated in the comic. The algorithm is as follows.
- Format the date and Dow Opening in this structure:
YYYY-MM-DD-DOW
. For example, it might look like2005-05-26-10458.68
. - Perform an md5 hash on the above. Remove any spacing or parsing characters, and split it in to two parts. For example, you might have these strings:
db9318c2259923d0
and8b672cb305440f97
. - Append each string to
0.
and convert to decimal. Using the above strings, we get the following:0.db9318c2259923d0
and0.8b672cb305440f97
, which is converted to decimal as aproximately0.8577132677070023444
and0.5445430695592821056
. You must truncate it down the the first 8 characters, producing0.857713
and0.544543
. - Combine the coordinates given through input and the decimals we just produced:
37
+0.857713
=37.857713
and-122
+0.544543
=-122.544543
Notes:
- To prevent the code from being unbearably long, you may use a built-in for the md5 hash.
- Standard loopholes (hardcoding, calling external resource) are forbidden.
May we take negative integers in the format
_#
instead of-#
, i.e._37
instead of-37
? – R. Kap – 2017-01-15T20:47:10.477Yes. As I stated, input and output can be flexible. – Julian Lachniet – 2017-01-15T22:55:13.707
3
Shame about not using built-ins. Python3's module antigravity would have works well https://hg.python.org/cpython/file/tip/Lib/antigravity.py
– george – 2017-01-16T10:51:56.213@george Did you make that module? – Julian Lachniet – 2017-01-16T16:27:33.253
@JulianLachniet Unfortunately not, never actually had a use for it though – george – 2017-01-16T16:42:05.910
@george Considering that module was created the day I posted the challenge, I'm a little bit suspicious. – Julian Lachniet – 2017-01-16T16:48:11.037
@JulianLachniet That page was only updated on the 16th, not created luckily. I've know about the modules for a few years. https://www.explainxkcd.com/wiki/index.php/353:_Python suggests that is as old as 2.7.0, which was released in 2010. https://www.python.org/download/releases/2.7/
– george – 2017-01-16T17:10:29.280@JulianLachniet That module has been around for a while. I used it in this answer more than a year ago (just to be funny, though.)
– mbomb007 – 2017-01-17T20:16:52.627