Mathematica 188 185 170 115 130 46 48 chars
Explanation
In earlier versions, I made a graph of positions having a chessboard distance of 1 from each other. GraphComponents
then revealed the number of islands, one per component.
The present version uses MorphologicalComponents
to find and number clusters of ones in the array--regions where 1
's are physically contiguous. Because graphing is unnecessary, this results in a huge economy of code.
Code
Max@MorphologicalComponents[#/.{"."->0,"*"->1}]&
Example
Max@MorphologicalComponents[#/.{"."->0,"*"->1}]&[{{".", ".", ".", ".", ".", ".", ".", ".", ".", "*", "*"}, {"*", "*", ".", ".", ".", ".", ".", ".", "*", "*", "*"}, {".", ".", ".", ".", ".", ".", ".", ".", ".", ".", "."}, {".", ".", ".", "*", ".", ".", ".", ".", ".", ".", "."}, {"*", ".", ".", ".", ".", ".", ".", ".", ".", "*", "."}, {"*", ".", ".", ".", ".", ".", ".", ".", ".", ".", "*"}}]
5
How it works
Data are input as an array; in Mathematica, this is a list of lists.
In the input array, data are converted to 1
's and 0
's by the replacement
/.{"."->0,"*"->1}
where /.
is an infix form of ReplaceAll
followed by replacement rules. This essentially converts the array into a black and white image. All we need to do is apply the function, Image
.
Image[{{".", ".", ".", ".", ".", ".", ".", ".", ".", "*", "*"}, {"*", "*", ".", ".", ".", ".", ".", ".", "*", "*", "*"}, {".", ".", ".", ".", ".", ".", ".", ".", ".", ".", "."}, {".", ".", ".", "*", ".", ".", ".", ".", ".", ".", "."}, {"*", ".", ".", ".", ".", ".", ".", ".", ".", "*", "."}, {"*", ".", ".", ".", ".", ".", ".", ".", ".", ".", "*"}} /. {"." -> 0, "*" -> 1}]

The white squares correspond to the cells having the value, 1.
The picture below shows a some steps the approach uses. The input matrix contains only 1
's and 0
's. The output matrix labels each morphological cluster with a number. (I wrapped both the input and output matrices in MatrixForm
to highlight their two dimensional structure.)
MorphologicalComponents
replaces 1
s with an integer corresponding to the cluster number of each cell.
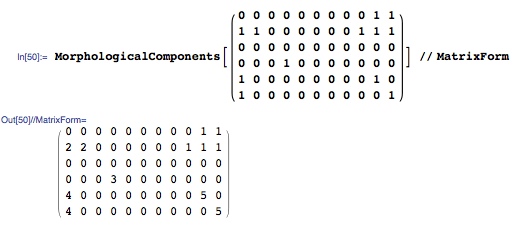
Max
returns the largest cluster number.
Displaying the Islands
Colorize
will color each island uniquely.
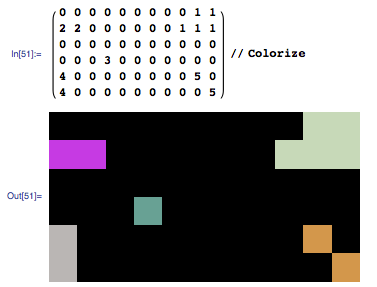
I don't understand the logic. Aren't 5 stars in the top right corner considered as one island? Then your example has 4 islands. – defhlt – 2012-08-14T04:42:08.023
the screen doesn't wrap. one island in each of the corners + the lone
*
island – Claudiu – 2012-08-14T04:59:55.3403But by your definition, an island is a group of '*' characters, implying more than one. – acolyte – 2012-08-14T20:09:06.827
oh fair point. stand-alone
*
s are also islands. – Claudiu – 2012-08-16T21:13:27.337