Mathematica
Rationale
Pi is an irrational number. It decimal representation does not repeat.
I strongly suspect that it does not "favor" any of the base ten digits, 0...9, nor any numbers of length when one partitions the decimal into strings of d digits. This suspicion can later be informally checked using the code below.
Code
The following will select integers between the integers x and y, where x is less than y.
y need not have the same number digits as x. The routine will begin at the cth digit of Pi. At the end of the procedure, the counter will be at c + n.
ClearAll[f]
f[x_,y_,c_,n_]:=
Module[{diff=y-x,d},
d=Length[IntegerDigits[diff]];
Cases[FromDigits/@Most[IntegerDigits[Round[N[Pi,c+n+1]10^(c+n-1)]]][[c;;c+n1]]~Partition~d,
i_/; i>x-1 && i<y+1]]
Suppose we want to generate a large (> 3000000), pseudo-random list of integers between 7 and
78. Here is how to request it. I've estimated that we'll need to use about 10^7 digits of Pi. We'll begin from position 1, that is, c=1.
(results= f[7,78,1, 10^7])//Timing
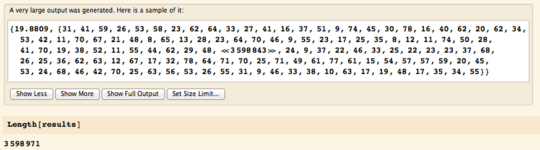
Note how the unsorted and untallied results maintain the order of Pi's digits: 314159...
Note also that the result took just under 20 seconds. As we move further along, we'll encounter practical limits to how much we can process in a reasonable amount of time. But since this is merely an exercise in thinking out of the box, we'll ignore this limitation as we proceed.
Examining the results
When we tally and plot the results we see that Pi does not particularly favor any numbers in this range:
SortBy[Tally[results],First]
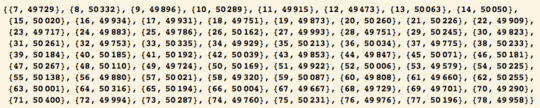
Notice that the integers between 7 and 78 were chosen more or less with equanimity. Further tests would show this holds up.
ListPlot[%]
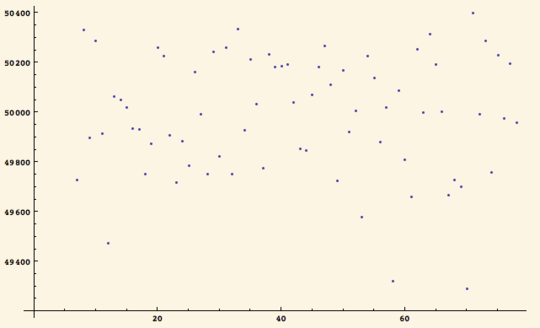
Similar checks should show whether or not Pi plays favorites with any numbers at all, regardless of how many digits it holds. [I checked for 1, 2, and 3 digit numbers over tens of millions of draws and found no bias starting from the beginning of Pi. A rigorous check would require more systematic examination or theoretical insights.]
1Are we supposed to generate a seemingly random number (or sequence of numbers) that can still be the same every time run, or do we need to get different numbers for each run. For the latter we positively need some source of an external seed that differs for each time run, which might be time or a file we've written to on our last run. – shiona – 2012-07-31T20:04:04.247
2Is this a golf or a challenge ? The question says golf but the tag says challenge ? – Paul R – 2012-07-31T22:07:42.380
1what is meant by an external source? – ardnew – 2012-08-01T01:31:55.287
@ardnew: Probably means that the program should run by itself, no webpages or files are referenced. – beary605 – 2012-08-01T17:10:27.557
What exactly constitutes a "random number seed"? Any constant, noise, or any other value that is used to generate any other number can be a "seed", Can you be more precise in your definition? Also, how pseudorandom must the generator be? – Joel Cornett – 2012-08-01T17:27:59.177
its a challenge, highest upvotes win. the random should be some way of doing it unique, and should change every time. Ultimately top votes win, so just have some fun with it and think outside of the box. – NRGdallas – 2012-08-01T18:28:07.293
alot of confusion on "external source" - the intent was that you couldn't use anything that is designed for random generation. As an example, time -could- be used, BUT its not really that original...
You just can't call random.org, or use something that basically does the job for you. – NRGdallas – 2012-08-01T18:30:48.147
@NRGdallas: Thanks. That cleared it up a lot. – Joel Cornett – 2012-08-01T21:44:47.563
3"Most votes" is not an objective winning criterion in the meaning of the FAQ. The winner should be obvious to a person examining the entries. Otherwise we have a simple popularity contest. – dmckee --- ex-moderator kitten – 2012-08-02T15:40:03.137
47 answers to a question that is apparently "not a real question". This site has so many negative people on it. Why does everything have to be objective? Photography competitions are subjective; is there not some art to solving many of these problems? – Griffin – 2012-08-02T15:42:12.163
6The SO asked the readers to judge submitted routines for a pseudo random number generator, taking into account the degree to which the proposals demonstrate original, "out of the box" thinking. This is admittedly subjective, but fully reasonable as a code challenge. The question is real. It is not ambiguous, vague, incomplete or rhetorical. It can be reasonably answered, as several submissions already made clear. It should not have been closed. – DavidC – 2012-08-02T16:39:19.960
@Griffin: Photography contests that allow public voting (as opposed to being voted on by an expert panel) are just as bad, as far as being a popularity contest goes. (I come from the view that popularity contests are content-free noise, and if you've seen the popularity contests that were all the rage back in the early days of Stack Overflow, you'll know what I'm talking about.) Next thing you know, people will want to post boat-programming questions here. Just say no. – Chris Jester-Young – 2012-08-02T19:04:32.170
@DavidCarraher: If you prefer, I'll be quite happy to choose a different close reason. The one I tend to use for "subjective winning criteria" questions is "off-topic". This is a matter of site policy: if you disagree with the policy, please make a post on meta where this can be discussed (although in the specific regard of the objectivity requirement, I believe this has been discussed multiple times before...). – Chris Jester-Young – 2012-08-02T19:09:36.207
1@ChrisJester-Young: I took your reason for closing the question at face value as a sincere claim. Apparently, you do not care what reason you give once you decide to close a question. By the way, reputations on Stack Exchange are officially based on the judgment of the community about the worth of contributions, so it's not as if this criterion were foreign to the enterprise. – DavidC – 2012-08-02T19:57:27.627
1@NRGdallas: If it's a challenge then please edit the word "golf" out of the question to avoid further confusion – Paul R – 2012-08-02T21:17:58.103
@DavidCarraher: (Apologies in advance if I misread your comment.) The list of close reasons is standard across the Stack Exchange network. There isn't a special close reason called "too subjective" just for this site; instead, it straddles the line between NARQ, Not Constructive, and Off-Topic. So you have to choose one. On reflection, Not Constructive is probably a better close reason than Off-Topic. But my point remains: questions that are not even the slightest bit objective are not generally a good fit for this site. The lack of a close reason tailored for that doesn't contradict my point. – Chris Jester-Young – 2012-08-02T22:06:27.493