x86 machine code, 9 7 bytes
D1 E9 SHR CX, 1 ; divide length in half
AD LODSW ; load next two chars into AH/AL
3A E0 CMP AH, AL ; compare AH and AL
E1 FB LOOPE -5 ; if equal, continue loop
Input string in SI
, input string length in CX
. Output ZF
if is double speak.
Or 14 bytes as a complete PC DOS executable:
B4 01 MOV AH, 01H ; DOS read char from STDIN (with echo)
CD 21 INT 21H ; read first char into AL
92 XCHG DX, AX ; put first char into DL
B4 08 MOV AH, 08H ; DOS read char from STDIN (no echo)
CD 21 INT 21H ; read second char into AL
3A C2 CMP AL, DL ; compare first and second char
74 F3 JE -13 ; if the same, continue loop
C3 RET ; otherwise exit to DOS
Input is via STDIN
, either pipe or interactive. Will echo the "de-doubled" input until a non-doubled character is detected, at which point will exit (maybe bending I/O rules a little bit, but this is just a bonus answer).
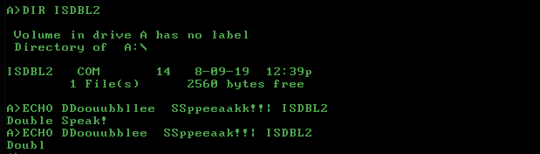
Build and test ISDBL2.COM using xxd -r
:
00000000: b401 cd21 92b4 08cd 213a c274 f3c3 ...!....!:.t..
Original 24 bytes complete PC DOS executable:
D1 EE SHR SI, 1 ; SI to DOS PSP (080H)
AD LODSW ; load string length into AL
D0 E8 SHR AL, 1 ; divide length in half
8A C8 MOV CL, AL ; put string length into BL
CLOOP:
AD LODSW ; load next two chars into AH/AL
3A E0 CMP AH, AL ; compare AH and AL
E1 FB LOOPE CLOOP ; if equal, continue loop
DONE:
B8 0E59 MOV AX, 0E59H ; BIOS tty function in AH, 'Y' in AL
74 02 JZ DISP ; if ZF, result was valid double
B0 4E MOV AL, 'N' ; if not, change output char to N
DISP:
B4 0E MOV AH, 0EH
CD 10 INT 10H
C3 RET ; return to DOS
Input from command line, output to screen 'Y'
if double, 'N'
if not.
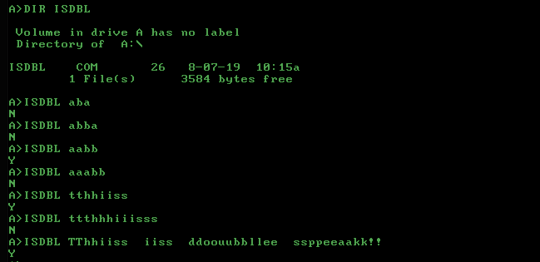
Build and test ISDBL.COM using xxd -r
:
00000000: d1ee add0 e88a c8ad 3ae0 e1fb b859 0e74 ........:....Y.t
00000010: 02b0 4eb4 0ecd 10c3 ..N.....
Credits:
1Can the Booleans or integers be (consistently) reversed? 1 for non-double speak, 0 for double speak – Luis Mendo – 2019-08-06T15:33:30.490
1@LuisMendo Unusual, but sounds okay to me. – AJFaraday – 2019-08-06T15:34:37.837
6May we error on inputs of length < 2? – cole – 2019-08-06T16:06:17.480
3Suggested test case:
abba
which should be falsey – Giuseppe – 2019-08-06T16:29:11.2772Suggested test case:
aabbbb
which should be truthy – Khuldraeseth na'Barya – 2019-08-06T17:30:04.397I guess correct spelling is not an issue, so something like "lleetteerr" would be considered truthy, right? Even though this could come from a program that says "double all characters except 't'". – Corak – 2019-08-07T06:21:41.530
1@Corak that’s correct. We’re not interested in the validity of words, just whether the characters all repeat. – AJFaraday – 2019-08-07T06:23:24.110
1Can I use exit code (0/1) to output? Standard I/O rules allow that. – val says Reinstate Monica – 2019-08-07T08:26:16.307
2@val Well, I'm not going to argue with standard I/O – AJFaraday – 2019-08-07T08:27:01.467
2Suggested test case:
0
which should be falsey. – 640KB – 2019-08-07T16:06:26.6371What about the empty string? – PieCot – 2019-08-07T21:04:17.770